Notification
React Bootstrap 5 Notification
React Bootstrap 5 notification is a component that allows you to send visitors to your website simple and easily customizable alert messages. Toasts are little notifications that are meant to look like the push notifications that have become popular on mobile and desktop platforms. Because they're made with flexbox, they're simple to align and place.
Importing the React Bootstrap 5 Notification Component
Start by importing CDBNotification
into your project to use the Contrast React Bootstrap 5 Notification component.
import { CDBNotification } from "cdbreact";
Custom Notification
Pro ComponentThere are a few options for the React Bootstrap 5 Notification. The show
option makes your notification component visible, while the fade
property makes your notification component fade out as it leaves your screen.
To alter the color of the icon in your notification, we add a text value to the iconClassName
prop on our CDBNotification
component, then attach the color we want it to have (e.g., primary, secondary, etc.). iconClassName = "text-primary" is an example
.
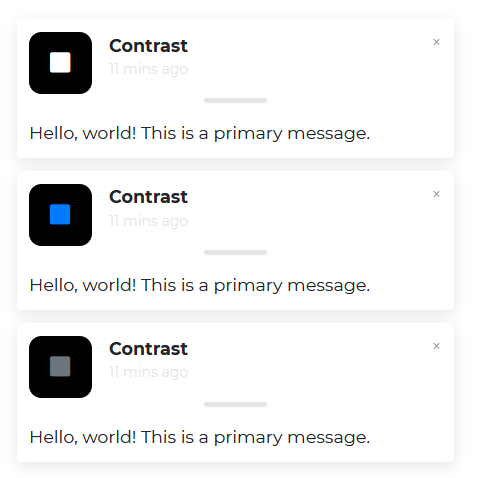
import React from "react";import { CDBNotification, CDBContainer } from "cdbreact";
export const Notification = () => { return ( <CDBContainer> <CDBNotification show fade autohide={2} title="Contrast" message="Hello, world! This is a primary message." text="11 mins ago" /> <CDBNotification show fade iconClassName="text-primary" title="Contrast" message="Hello, world! This is a primary message." text="11 mins ago" /> <CDBNotification show fade iconClassName="text-secondary" title="Contrast" message="Hello, world! This is a primary message." text="11 mins ago" /> </CDBContainer> );};
Notification with Hidden Message
Pro Component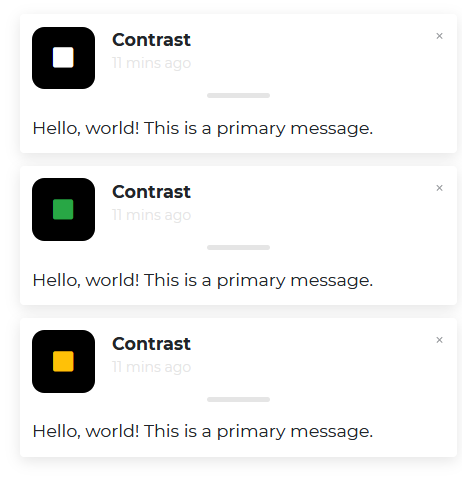
import React from "react";import { CDBNotification, CDBContainer } from "cdbreact";
export const Notification = () => { return ( <CDBContainer> <CDBNotification show fade hideMessage autohide={5} title="Contrast" message="Hello, world! This is a primary message." text="11 mins ago" /> <CDBNotification show fade hideMessage iconClassName="text-success" title="Contrast" message="Hello, world! This is a primary message." text="11 mins ago" /> <CDBNotification show fade hideMessage iconClassName="text-warning" title="Contrast" message="Hello, world! This is a primary message." text="11 mins ago" /> </CDBContainer> );};
Notification with Icons
Pro ComponentYou can customize the icon on your notification using the Contrast React Bootstrap 5 Notification component. Use the icon
prop to do so.
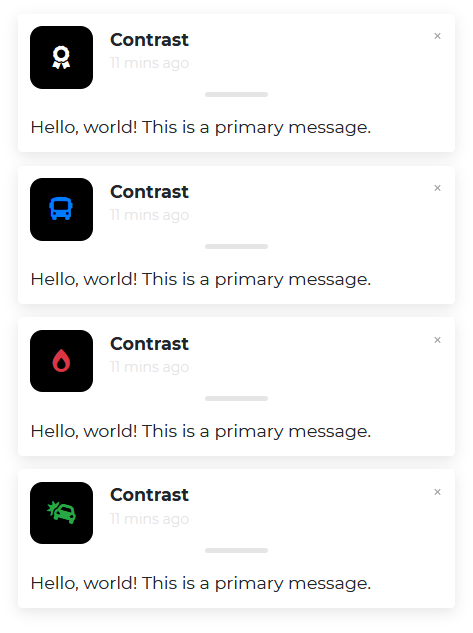
import React from "react";import { CDBNotification, CDBContainer } from "cdbreact";
export const Notification = () => { return ( <CDBContainer> <CDBNotification show fade title="Contrast" icon="award" message="Hello, world! This is a primary message." text="11 mins ago" /> <CDBNotification show fade iconClassName="text-primary" title="Contrast" icon="bus" message="Hello, world! This is a primary message." text="11 mins ago" />
<CDBNotification show fade iconClassName="text-danger" title="Contrast" icon="burn" message="Hello, world! This is a primary message." text="11 mins ago" /> <CDBNotification show fade iconClassName="text-success" icon="car-crash" title="Contrast" message="Hello, world! This is a primary message." text="11 mins ago" /> </CDBContainer> );};
Positioning
Pro ComponentThe position
parameter specifies where the notification should appear on the webpage.
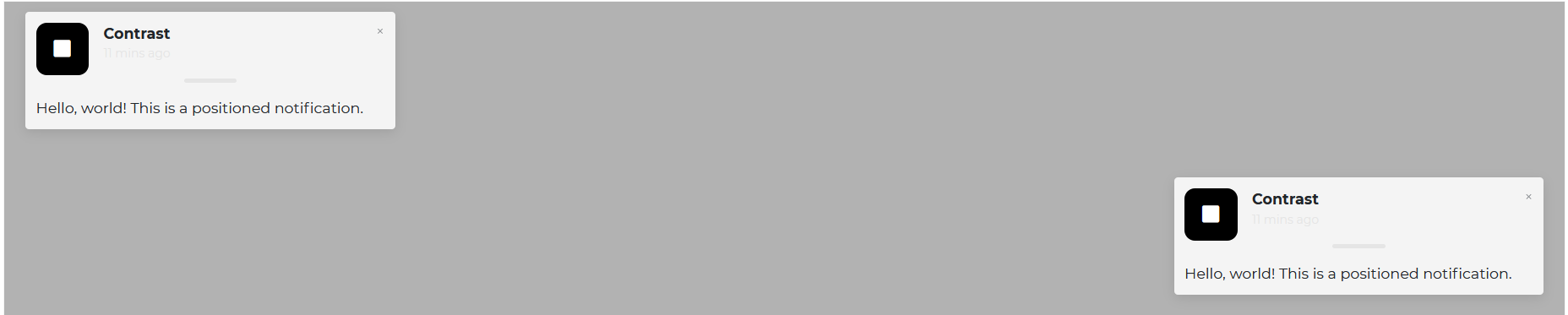
import React from "react";import { CDBNotification, CDBContainer } from "cdbreact";
export const Notification = () => { return ( <CDBContainer className="position-relative"> <CDBNotification show fade title="Contrast" message="Hello, world! This is a positioned notification." text="11 mins ago" position="top-left" /> <CDBNotification show fade title="Contrast" message="Hello, world! This is a positioned notification." text="11 mins ago" position="bottom-right" /> </CDBContainer> );};
Contrast React Bootstrap 5 Notification Props
The props you get to use with the React Bootstrap 5 Notification component will be elaborated upon in this section. You'll learn what these props do, how to utilize them in your code, and what their default values is.
Other prop settings for the 'CDBNotification' component are listed in the table below.
Name | Type | Default | Description | Example |
---|---|---|---|---|
bodyClassName | String | Adds custom className to the body section of notification component | <CDBNotification bodyClassName="p-5 font-weight-bold" .../> | |
bodyColor | String | Set color to the body section of notification component | <CDBNotification bodyColor="black" .../> | |
ClassName | String | Adds custom classes to the wrapper | <CDBNotification ClassName="stylish-color-dark" .../> | |
closeClassName | String | text-dark | Adds custom classes to the Close button | <CDBNotification closeClassName="blue-grey-text" .../> |
tag | function or String | div | Changes default tag | <CDBNotification tag="div" ... /> |
fade | Boolean | false | Adds a fade effect when you close the notification | <CDBNotification fade .../> |
hideMessage | Boolean | false | Hides messages in the component | <CDBNotification hideMessage .../> |
autohide | Number | Sets time in ms when box hide. 0 = infinity | <CDBNotification autoHide={3000} .../> | |
icon | String | square | Sets a custom icon | <CDBNotification icon="bell" ... /> |
iconClassName | String | Adds custom classes to the Icon | <CDBNotification iconClassName="green-text" ... /> | |
message | String | Sets message of the notification | <CDBNotification message="Hello there! This is a toast message" ... /> | |
position | String | One of top-right, top-center, top-left, bottom-right, bottom-center, bottom-left | <CDBNotification position="top-right" ... /> | |
show | Boolean | false | Shows your component. If you do not use this setting, your component will exist, but it will be hidden. | <CDBNotification show .../> |
text | String | Sets text of the notification | <CDBNotification text="11 minutes ago" ... /> | |
title | String | Set header text of the notification | <CDBNotification title="Someone sent you an email" ... /> | |
titleClassName | String | Adds custom classes to the title text | <CDBNotification titleClassName="elegant-color-dark-white-text" ... /> | |
titleColor | String | Sets the color of the title | <CDBNotification titleColor="white" ... /> |
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.