Charts
React Bootstrap 5 Charts
React Bootstrap 5 Charts are pictorial representation or diagrams that showcases data or values in an organized manner. CDBReact Bootstrap charts have easy-to-use syntax, responsive and flexible. There are twelve types of charts available for your use. To gain more insight on how to use the charts check out react chartsjs.
Line Chart
import React, { useState } from 'react';import { Line } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Eating', 'Drinking', 'Sleeping', 'Designing', 'Coding', 'Cycling', 'Running'], datasets: [ { label: 'My First dataset', backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', data: [65, 59, 90, 81, 56, 55, 40], }, { label: 'My Second dataset', backgroundColor: 'rgba(71, 225, 167, 0.5)', borderColor: 'rgb(71, 225, 167)', data: [28, 48, 40, 19, 96, 27, 100], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Line chart</h3> <Line data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Dynamically Refreshed Line chart
import React, { useState, useEffect } from 'react';import { Line } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data, setData] = useState({ labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'], datasets: [ { label: 'My First dataset', fill: false, lineTension: 0.1, backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', borderCapStyle: 'butt', borderDash: [], borderDashOffset: 0.0, borderJoinStyle: 'miter', pointBorderColor: 'rgba(75,192,192,1)', pointBackgroundColor: '#fff', pointBorderWidth: 1, pointHoverRadius: 5, pointHoverBackgroundColor: 'rgba(71, 225, 167, 0.5)', pointHoverBorderColor: 'rgb(71, 225, 167)', pointHoverBorderWidth: 2, pointRadius: 1, pointHitRadius: 10, data: [65, 59, 80, 81, 56, 55, 40], }, ], });
useEffect(() => { setInterval(function() { var oldDataSet = data.datasets[0]; var newData = [];
for (var x = 0; x < data.labels.length; x++) { newData.push(Math.floor(Math.random() * 100)); }
var newDataSet = { ...oldDataSet, };
newDataSet.data = newData;
var newState = { ...data, datasets: [newDataSet], };
setData(newState); }, 5000); }, []);
return ( <CDBContainer> <h3 className="mt-5">Dynamicly Refreshed Line chart</h3> <Line data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Scatter Chart
import React, { useState } from 'react';import { Scatter } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Bubble'], datasets: [ { label: 'My First dataset', fill: false, lineTension: 0.1, backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', borderCapStyle: 'butt', borderDash: [], borderDashOffset: 0.0, borderJoinStyle: 'miter', pointBorderColor: 'rgba(194, 116, 161, 0.5)', pointBackgroundColor: '#fff', pointBorderWidth: 1, pointHoverRadius: 5, pointHoverBackgroundColor: 'rgba(75,192,192,1)', pointHoverBorderColor: 'rgba(220,220,220,1)', pointHoverBorderWidth: 2, pointRadius: 1, pointHitRadius: 10, data: [ { x: 65, y: 75 }, { x: 75, y: 12 }, { x: 89, y: 54 }, { x: 94, y: 23 }, { x: 59, y: 49 }, { x: 80, y: 90 }, { x: 81, y: 29 }, { x: 56, y: 36 }, { x: 55, y: 25 }, { x: 40, y: 18 }, ], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Scatter chart</h3> <Scatter data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Bubble Chart
import React, { useState } from 'react';import { Bubble } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Bubble'], datasets: [ { label: 'My First dataset', fill: false, lineTension: 0.1, backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', borderCapStyle: 'butt', borderDash: [], borderDashOffset: 0.0, borderJoinStyle: 'miter', pointBorderColor: 'rgba(194, 116, 161, 0.5)', pointBackgroundColor: '#fff', pointBorderWidth: 1, pointHoverRadius: 5, pointHoverBackgroundColor: 'rgba(75,192,192,1)', pointHoverBorderColor: 'rgba(220,220,220,1)', pointHoverBorderWidth: 2, pointRadius: 1, pointHitRadius: 10, data: [ { x: 65, y: 75 }, { x: 75, y: 12 }, { x: 89, y: 54 }, { x: 94, y: 23 }, { x: 59, y: 49 }, { x: 80, y: 90 }, { x: 81, y: 29 }, { x: 56, y: 36 }, { x: 55, y: 25 }, { x: 40, y: 18 }, ], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Bubble chart</h3> <Bubble data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Bar Chart
import React, { useState } from 'react';import { Bar } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Eating', 'Drinking', 'Sleeping', 'Designing', 'Coding', 'Cycling', 'Running'], datasets: [ { label: 'My First dataset', backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', data: [65, 59, 90, 81, 56, 55, 40], }, { label: 'My Second dataset', backgroundColor: 'rgba(71, 225, 167, 0.5)', borderColor: 'rgb(71, 225, 167)', data: [28, 48, 40, 19, 96, 27, 100], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Bar chart</h3> <Bar data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Horizontal Bar Chart
import React, { useState } from 'react';import { HorizontalBar } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Eating', 'Drinking', 'Sleeping', 'Designing', 'Coding', 'Cycling', 'Running'], datasets: [ { label: 'My First dataset', backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', data: [65, 59, 90, 81, 56, 55, 40], }, { label: 'My Second dataset', backgroundColor: 'rgba(71, 225, 167, 0.5)', borderColor: 'rgb(71, 225, 167)', data: [28, 48, 40, 19, 96, 27, 100], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Horizontal Bar chart</h3> <HorizontalBar data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Dynamically Refreshed Bar Chart
import React, { useState, useEffect } from 'react';import { Bar } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data, setData] = useState({ labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'], datasets: [ { label: 'My First dataset', fill: false, lineTension: 0.1, backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', borderCapStyle: 'butt', borderDash: [], borderDashOffset: 0.0, borderJoinStyle: 'miter', pointBorderColor: 'rgba(75,192,192,1)', pointBackgroundColor: '#fff', pointBorderWidth: 1, pointHoverRadius: 5, pointHoverBackgroundColor: 'rgba(71, 225, 167, 0.5)', pointHoverBorderColor: 'rgb(71, 225, 167)', pointHoverBorderWidth: 2, pointRadius: 1, pointHitRadius: 10, data: [65, 59, 80, 81, 56, 55, 40], }, ], });
useEffect(() => { setInterval(function() { var oldDataSet = data.datasets[0]; var newData = [];
for (var x = 0; x < data.labels.length; x++) { newData.push(Math.floor(Math.random() * 100)); }
var newDataSet = { ...oldDataSet, };
newDataSet.data = newData;
var newState = { ...data, datasets: [newDataSet], };
setData(newState); }, 5000); }, []);
return ( <CDBContainer> <h3 className="mt-5">Dynamicly Refreshed Bar chart</h3> <Bar data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Pie Chart
import React, { useState } from 'react';import { Pie } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Eating', 'Drinking', 'Sleeping', 'Designing', 'Coding', 'Cycling', 'Running'], datasets: [ { label: 'My First dataset', backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', data: [65, 59, 90, 81, 56, 55, 40], }, { label: 'My Second dataset', backgroundColor: 'rgba(71, 225, 167, 0.5)', borderColor: 'rgb(71, 225, 167)', data: [28, 48, 40, 19, 96, 27, 100], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Pie chart</h3> <Pie data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Doughnut Chart
import React, { useState } from 'react';import { Doughnut } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Eating', 'Drinking', 'Sleeping', 'Designing', 'Coding', 'Cycling', 'Running'], datasets: [ { label: 'My First dataset', backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', data: [65, 59, 90, 81, 56, 55, 40], }, { label: 'My Second dataset', backgroundColor: 'rgba(71, 225, 167, 0.5)', borderColor: 'rgb(71, 225, 167)', data: [28, 48, 40, 19, 96, 27, 100], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Doughnut chart</h3> <Doughnut data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Dynamically Refreshed Doughnut Chart
import React, { useState, useEffect } from 'react';import { Doughnut } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data, setData] = useState({ labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'], datasets: [ { label: 'My First dataset', fill: false, lineTension: 0.1, backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', borderCapStyle: 'butt', borderDash: [], borderDashOffset: 0.0, borderJoinStyle: 'miter', pointBorderColor: 'rgba(75,192,192,1)', pointBackgroundColor: '#fff', pointBorderWidth: 1, pointHoverRadius: 5, pointHoverBackgroundColor: 'rgba(71, 225, 167, 0.5)', pointHoverBorderColor: 'rgb(71, 225, 167)', pointHoverBorderWidth: 2, pointRadius: 1, pointHitRadius: 10, data: [65, 59, 80, 81, 56, 55, 40], }, ], });
useEffect(() => { setInterval(function() { var oldDataSet = data.datasets[0]; var newData = [];
for (var x = 0; x < data.labels.length; x++) { newData.push(Math.floor(Math.random() * 100)); }
var newDataSet = { ...oldDataSet, };
newDataSet.data = newData;
var newState = { ...data, datasets: [newDataSet], };
setData(newState); }, 5000); }, []);
return ( <CDBContainer> <h3 className="mt-5">Dynamicly Refreshed Doughnut chart</h3> <Doughnut data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Polar Chart
import React, { useState } from 'react';import { Polar } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Eating', 'Drinking', 'Sleeping', 'Designing', 'Coding', 'Cycling', 'Running'], datasets: [ { label: 'My First dataset', backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', data: [65, 59, 90, 81, 56, 55, 40], }, { label: 'My Second dataset', backgroundColor: 'rgba(71, 225, 167, 0.5)', borderColor: 'rgb(71, 225, 167)', data: [28, 48, 40, 19, 96, 27, 100], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Polar chart</h3> <Polar data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Radar Chart
import React, { useState } from 'react';import { Radar } from 'react-chartjs-2';import { CDBContainer } from 'cdbreact';
const Chart = () => { const [data] = useState({ labels: ['Eating', 'Drinking', 'Sleeping', 'Designing', 'Coding', 'Cycling', 'Running'], datasets: [ { label: 'My First dataset', backgroundColor: 'rgba(194, 116, 161, 0.5)', borderColor: 'rgb(194, 116, 161)', data: [65, 59, 90, 81, 56, 55, 40], }, { label: 'My Second dataset', backgroundColor: 'rgba(71, 225, 167, 0.5)', borderColor: 'rgb(71, 225, 167)', data: [28, 48, 40, 19, 96, 27, 100], }, ], });
return ( <CDBContainer> <h3 className="mt-5">Radar chart</h3> <Radar data={data} options={{ responsive: true }} /> </CDBContainer> );};
export default Chart;
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
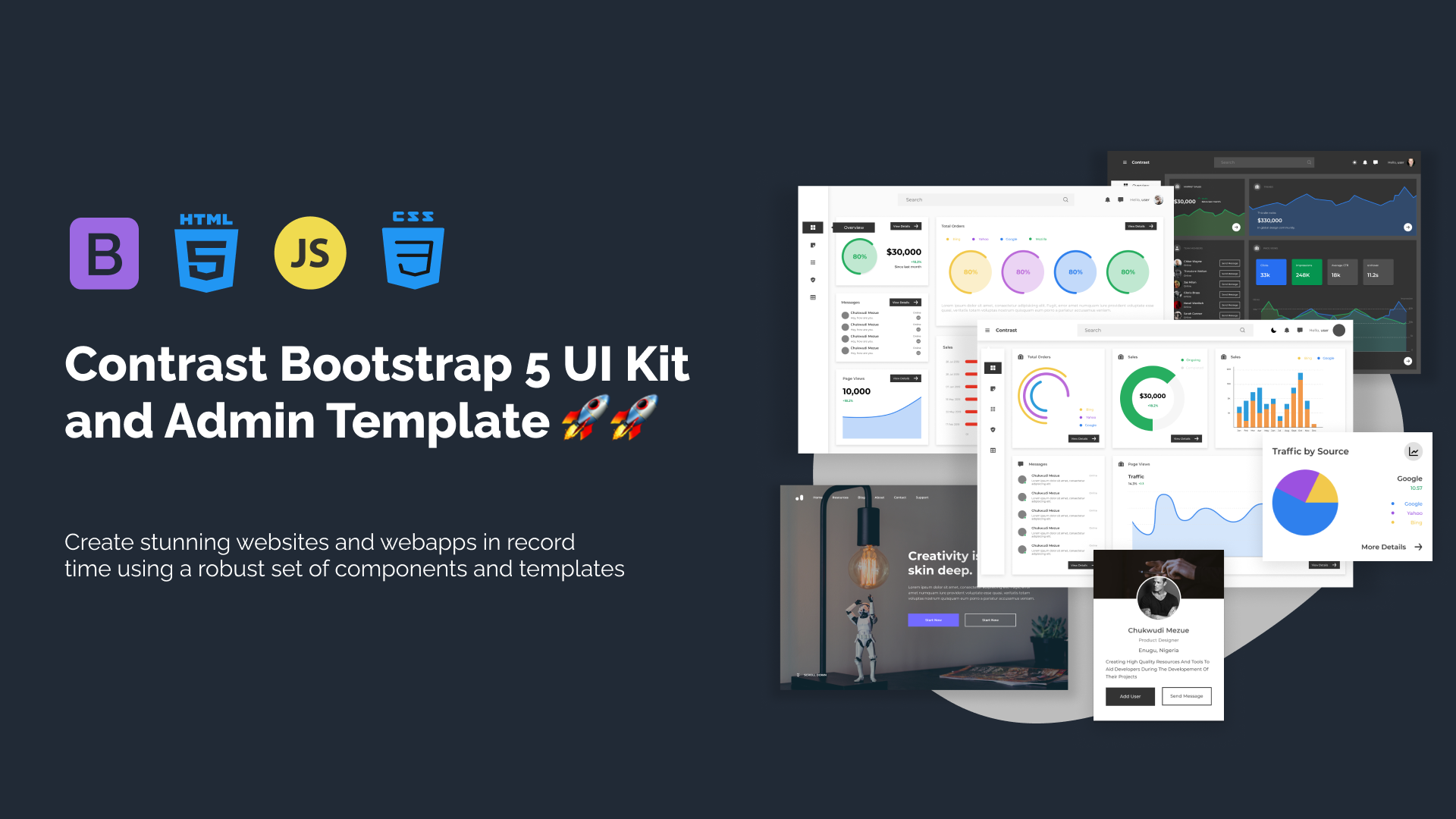