Tutorial: Javascript Math Object
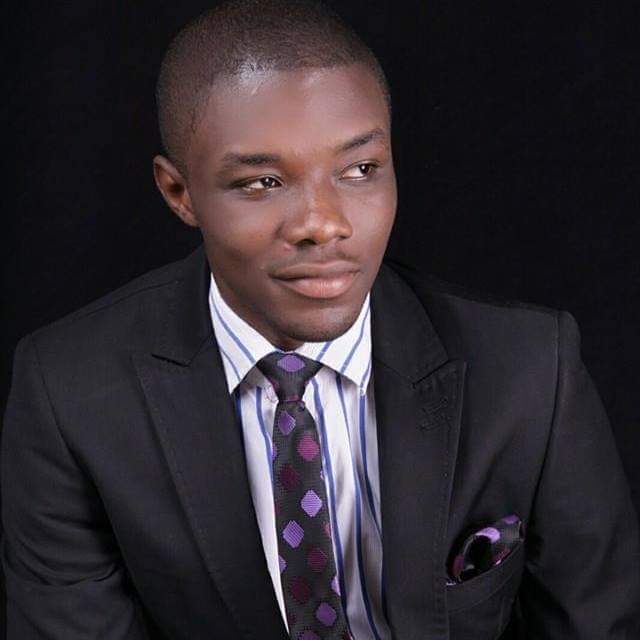
By Emmanuel Chinonso
Web Developer
JavaScript Math Object
The JavaScript Math object lets you do math using numbers.
JavaScript Code:
Math.PI; // returns 3.141592653589793
The Math Object
The Math object, unlike other objects, does not have a constructor. The Math object is a one-dimensional object. Without first constructing a Math object, all methods and properties are available.
Math Properties (Constants)
Math.property is the syntax for any Math property. Math properties in JavaScript allow access to eight mathematical constants:
JavaScript Code:
Math.E; // returns Euler's numberMath.PI; // returns PIMath.SQRT2; // returns the square root of 2Math.SQRT1_2; // returns the square root of 1/2Math.LN2; // returns the natural logarithm of 2Math.LN10; // returns the natural logarithm of 10Math.LOG2E; // returns base 2 logarithm of EMath.LOG10E; // returns base 10 logarithm of E
Math Methods
The syntax for all Math methods is as follows: Math.method.(number) Integer to Number To round a number to an integer, there are four popular methods: Math.round(x) Returns the value of x rounded to the closest integer. Math.ceil(x) Returns the value of x, rounded to the closest integer. Math.floor(x) The value of x is rounded down to the nearest integer. Math.trunc(x) The integer component of x is returned (new in ES6) Math.round() Math.round(x) produces the integer closest to the given value
JavaScript Code:
Math.round(4.9); // returns 5Math.round(4.7); // returns 5Math.round(4.4); // returns 4Math.round(4.2); // returns 4Math.round(-4.2); // returns -4Math.ceil();
The value of x rounded up to the nearest integer is returned by Math.ceil(x):
JavaScript Code:
Math.ceil(4.9); // returns 5Math.ceil(4.7); // returns 5Math.ceil(4.4); // returns 5Math.ceil(4.2); // returns 5Math.ceil(-4.2); // returns -4Math.floor();
The value of x reduced down to the closest integer is returned by Math.floor(x):
JavaScript Code:
Math.floor(4.9); // returns 4Math.floor(4.7); // returns 4Math.floor(4.4); // returns 4Math.floor(4.2); // returns 4Math.floor(-4.2); // returns -5Math.trunc();
Math.trunc(x) returns the integer part of x:
JavaScript Code:
Math.trunc(4.9); // returns 4Math.trunc(4.7); // returns 4Math.trunc(4.4); // returns 4Math.trunc(4.2); // returns 4Math.trunc(-4.2); // returns -4
JavaScript Random
Math.random()
Math.random() returns a random number between 0 (inclusive), and 1 (exclusive):
JavaScript Code:
Math.random(); // returns a random number
Math.random() always produces a value that is less than 1.
JavaScript Random Integers
Random integers can be returned using Math.random() and Math.floor().
JavaScript Code:
Math.floor(Math.random() * 10); // returns a random integer from 0 to 9Math.floor(Math.random() * 11); // returns a random integer from 0 to 10Math.floor(Math.random() * 100); // returns a random integer from 0 to 99Math.floor(Math.random() * 101); // returns a random integer from 0 to 100Math.floor(Math.random() * 10) + 1; // returns a random integer from 1 to 10Math.floor(Math.random() * 100) + 1; // returns a random integer from 1 to 100.
A Proper Random Function
As you can see from the examples above, creating a suitable random function to utilize for all random integer uses could be a smart idea. This JavaScript function always returns a number between min (included) and max (excluded) that is between min (included) and max (excluded):
JavaScript Code:
function getRndInteger(min, max) { return Math.floor(Math.random() * (max - min)) + min;}
This JavaScript function always produces a number between min and max (both included) that is random:
JavaScript Code:
function getRndInteger(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min;}
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts