Tutorial: Javascript Date Objects
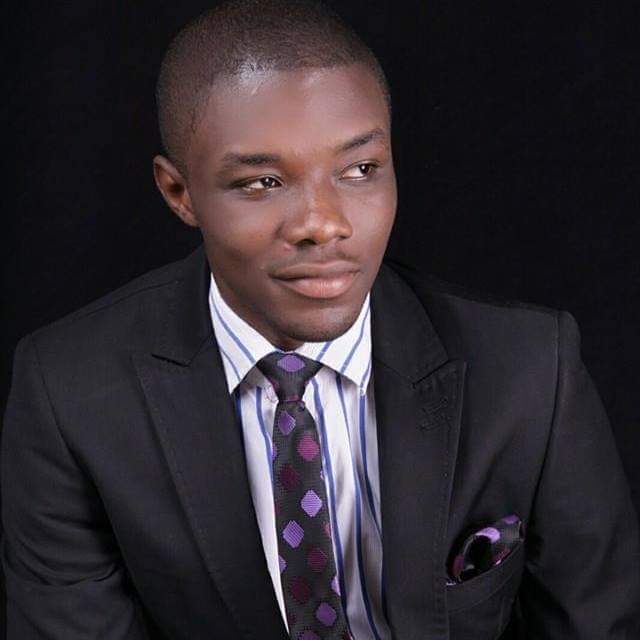
By Emmanuel Chinonso
Web Developer
JavaScript Date Objects
The JavaScript Date Object allows us to manipulate dates. By default, JavaScript will display a date as a full text string using the browser's time zone.
The new Date() constructor is used to build date objects. A new date object can be made in four ways:
- new Date()
- new Date(year, month, day, hours, minutes, seconds, milliseconds)
- new Date(milliseconds)
- new Date(date string)
new Date(): new Date() creates a date object that contains the current date and time:
var d = new Date();
Date objects are always the same. Although the computer clock ticks, date objects do not.
- new Date(year, month, ...)
- new Date(year, month, ...) generates a new date object with the date and time supplied.
Year, month, day, hour, minute, second, and millisecond (in that sequence) are specified by seven numbers:
var d = new Date(2018, 11, 24, 10, 33, 30, 0);
From 0 to 11 months are counted in JavaScript. The month of January has a value of 0. The year, month, day, hour, minute, and second are all represented by six numbers: year, month, day, hour, minute, and second.
var d = new Date(2018, 11, 24, 10, 33, 30);
JavaScript Stores Dates as Milliseconds
Since January 1, 1970, at 00:00:00 UTC, JavaScript has stored dates as a number of milliseconds (Universal Time Coordinated). The time is 00:00:00 UTC on January 1, 1970.
The current date and time is: January 1, 1970, milliseconds ago.
Date Methods
When you create a Date object, you can use a variety of methods to manipulate it. The year, month, day, hour, minute, second, and millisecond of date objects can be retrieved and set using either local or UTC (universal, or GMT) time.
Displaying Dates
By default, JavaScript outputs dates in full text string format: 01:00:00 GMT+0100 on Wednesday, March 25th, 2015 (West Africa Standard Time) When you display a date object in HTML, the toString() method converts it to a string automatically.
d = new Date();document.getElementById('demo').innerHTML = d;
Same as:
d = new Date();document.getElementById('demo').innerHTML = d.toString();
To convert a date to a UTC string, use the toUTCString() function (a date display standard).
var d = new Date();document.getElementById('demo').innerHTML = d.toUTCString();
To convert a date to a more readable format, use the toDateString() method:
var d = new Date();document.getElementById('demo').innerHTML = d.toDateString();
The toISOString() method uses the ISO standard format to convert a date to a string:
var d = new Date();document.getElementById('demo').innerHTML = d.toISOString();
JavaScript Get Date Methods
To get data from a date object, use these methods:
Method | Description |
---|---|
getFullYear() | Get the year as a four digit number (yyyy) |
getMonth() | Get the month as a number (0-11) |
getDate() | Get the day as a number (1-31) |
getHours() | Get the hour (0-23) |
getMinutes() | Get the minute (0-59) |
getSeconds() | Get the second (0-59) |
getMilliseconds() | Get the millisecond (0-999) |
getTime() | Get the time (milliseconds since January 1, 1970) |
getDay() | Get the weekday as a number (0-6) |
Date.now() | Get the time. ECMAScript 5. |
The getTime() Method: Since January 1, 1970, the getTime() method returns the following value in milliseconds:
var d = new Date();document.getElementById("demo").innerHTML = d.getTime();The getFullYear() MethodThe getFullYear() method returns the year of a date as a four digit number:
var d = new Date();document.getElementById("demo").innerHTML = d.getFullYear();
The getDate() Method: The getDate() method produces a number (1-31) representing the day of a date:
var d = new Date();document.getElementById('demo').innerHTML = d.getDate();
The getHours() Method: The getHours() method returns a number (0-23) representing the hours of a date:
var d = new Date();document.getElementById('demo').innerHTML = d.getHours();
The getMinutes() Method: The getMinutes() method returns a number (0-59) representing the minutes of a date:
var d = new Date();document.getElementById('demo').innerHTML = d.getMinutes();
The getSeconds() Method: The getSeconds() method returns a number (0-59) representing the seconds of a date:
var d = new Date();document.getElementById('demo').innerHTML = d.getSeconds();
The getMilliseconds() Method: The getMilliseconds() method returns the milliseconds of a date as a number (0-999):
var d = new Date();document.getElementById('demo').innerHTML = d.getMilliseconds();
The getDay() Method: The getDay() method produces a number (0-6) representing the weekday of a date:
var d = new Date();document.getElementById('demo').innerHTML = d.getDay();
Even though some nations consider the first day of the week to be "Monday," the first day of the week (0) in JavaScript implies "Sunday." You can return the weekday as a name using an array of names and getDay():
var d = new Date();var days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'];document.getElementById('demo').innerHTML = days[d.getDay()];
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts