Tutorial: Javascript Objects
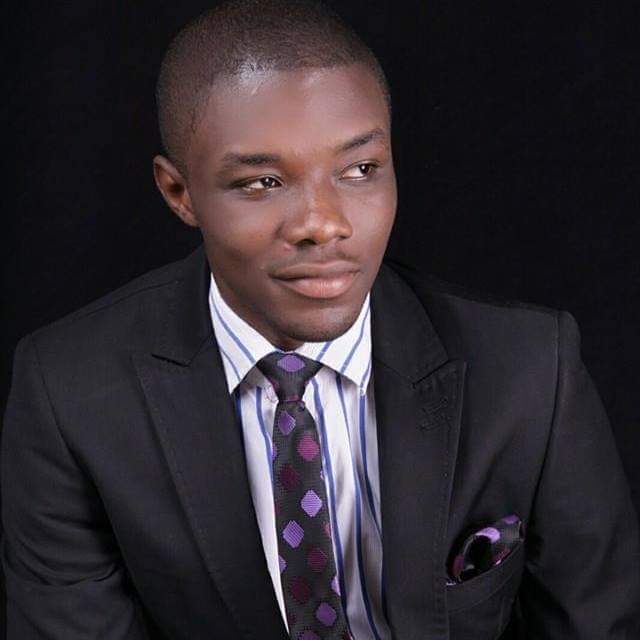
By Emmanuel Chinonso
Web Developer
JavaScript Object
A automobile is an object in real life. A car has properties such as weight and color, as well as procedures such as start and stop. All cars have the same properties, yet the values of those properties vary from one to the next. The processes are the identical in all cars, however they are conducted at different times.
JavaScript variables, as you undoubtedly know, are containers for data values. Objects are also variables. Objects, on the other hand, might have a wide range of values. Name: value pairs are used to represent the values (name and value separated by a colon). JavaScript objects are containers for named values that are referred to as properties or methods.
Object Definition
An object literal is used to define (and construct) a JavaScript object:
JavaScript Code:
var person = { firstName: 'Johnny', lastName: 'Williams', age: 35, eyeColor: 'brown',};
It is not necessary to use spaces or line breaks. Multiple lines can be used to define an object.
Object Properties
In JavaScript objects, The name: value pairs are referred to as properties:
Property | Property Value |
---|---|
firstName | Johnny |
------- | -------- |
lastName | Williams |
------- | -------- |
age | 35 |
------- | -------- |
eyeColor | brown |
Accessing Object Properties
Object properties can be accessed in two ways:
objectName.propertyName
or
objectName["propertyName"]
JavaScript Code:
person.lastName;
Or
person['lastName'];
Object Methods Methods can be added to objects. Actions that can be done on objects are referred to as methods. Methods are stored as function definitions in properties.
Property | Property Value |
---|---|
firstName | Johnny |
------- | -------- |
lastName | Williams |
------- | -------- |
age | 35 |
------- | -------- |
eyeColor | brown |
------- | -------- |
fullName | function () {return this.firstName + " " + this.lastName;} |
A property that stores a function is known as a method.
JavaScript Code:
var person = { firstName: 'John', lastName: 'Doe', id: 5566, fullName: function() { return this.firstName + ' ' + this.lastName; },};
The this Keyword
This
refers to the "owner" of a function in a function definition. This
is the object that "owns" the fullName function in the previous example. To put it another way: The firstName property of this object is referred to as firstName.
Object Methods Are Accessible
The following is the syntax for calling an object method:
objectName.methodName()
JavaScript Code:
name = person.fullName();
If you call a method without the () parenthesis, the function definition is returned:
JavaScript Code:
name = person.fullName;
Strings, Numbers, and Booleans should not be declared as objects!
When the keyword "new" is used to declare a JavaScript variable, it creates the variable as an object:
JavaScript Code:
var x = new String(); // Declares x as a String objectvar y = new Number(); // Declares y as a Number objectvar z = new Boolean(); // Declares z as a Boolean object
String, Number, and Boolean objects should all be avoided at all costs. They make your code more difficult to understand and slow down the speed with which it runs.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts