Tutorial: Sorting In Javascript
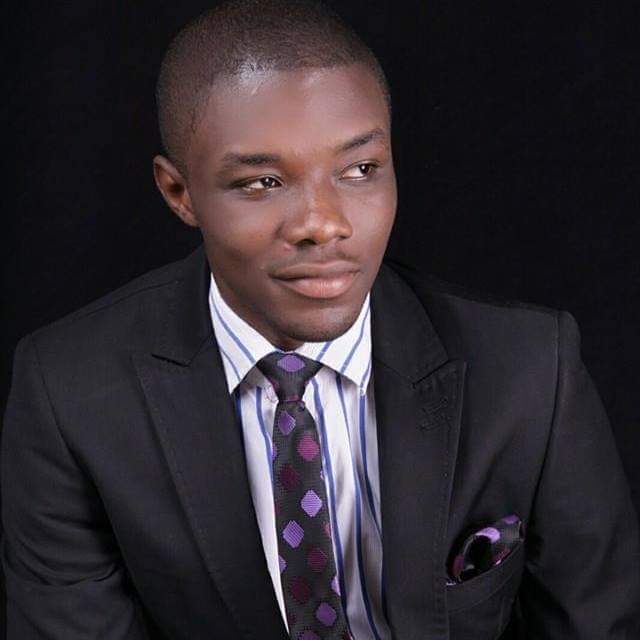
By Emmanuel Chinonso
Web Developer
JavaScript Sorting Arrays
Sorting an Array
The sort() method alphabetically sorts an array:
JavaScript Code:
var fruits = ['Banana', 'Orange', 'Apple', 'Mango'];fruits.sort(); // Sorts the elements of fruits
Reversing an Array The reverse() method reverses an array's elements. It can be used to sort an array in descending order as follows:
JavaScript Code:
var fruits = ['Banana', 'Orange', 'Apple', 'Mango'];fruits.sort(); // First sort the elements of fruitsfruits.reverse(); // Then reverse the order of the elements
Numeric Sort: The sort() function arranges data as strings by default. This is great for strings ("Apple" comes before "Banana"). When numbers are arranged as strings, however, "25" is more than "100," because "2" is greater than "1." As a result, when sorting numbers, the sort() method will return an inaccurate result. This can be remedied by including a comparison function:
JavaScript Code:
var points = [40, 100, 1, 5, 25, 10];points.sort(function(a, b) { return a - b;});
Use the same trick to sort an array descending:
JavaScript Code:
var points = [40, 100, 1, 5, 25, 10];points.sort(function(a, b) { return b - a;});
The Compare Function The comparison function's goal is to define a different sort order. Depending on the arguments, the comparison function should return a negative, zero, or positive value:
function(a, b){return a - b}
When the sort() method compares two numbers, it passes them to the compare function, which sorts them based on the (negative, zero, positive) value returned. If the outcome is negative, an is ranked first, followed by b. If the outcome is positive, b is ranked first, followed by a. If the result is 0, no modifications to the two values' sort order are made.
Example: The compare function compares two values at a time throughout the entire array (a, b). The sort() method uses the compare function to compare 40 and 100. (40, 100). The sort function sorts 40 as a number less than 100 since the function calculates 40 - 100 (a - b) and the result is negative (-60). You may play about with numerical and alphabetical sorting using this code snippet:
<button onclick="myFunction1()">Sort Alphabetically</button><button onclick="myFunction2()">Sort Numerically</button><p id="demo"></p>
<script> var points = [40, 100, 1, 5, 25, 10]; document.getElementById("demo").innerHTML = points; function myFunction1() { points.sort(); document.getElementById("demo").innerHTML = points; } function myFunction2() { points.sort(function(a, b){return a - b}); document.getElementById("demo").innerHTML = points; }</script>
Sorting an Array in Random Order
JavaScript Code:
var points = [40, 100, 1, 5, 25, 10];points.sort(function(a, b) { return 0.5 - Math.random();});
The Fisher Yates Method
The array.sort() function in the above code is not accurate; it favors some values over others. The Fisher Yates shuffle is the most popular right method, and it was first used in data science in 1938! The method can be converted into JavaScript as follows:
JavaScript Code:
var points = [40, 100, 1, 5, 25, 10];for (i = points.length - 1; i > 0; i--) { j = Math.floor(Math.random() * i); k = points[i]; points[i] = points[j]; points[j] = k;}
Find the Highest (or Lowest) Array Value
In an array, there are no built-in functions for finding the maximum or minimum value. You can use the index to get the highest and lowest values after you've sorted an array.
Sorting ascending:
JavaScript Code:
var points = [40, 100, 1, 5, 25, 10];points.sort(function(a, b) { return a - b;});// now points[0] contains the lowest value// and points[points.length-1] contains the highest value
Sorting descending:
JavaScript Code:
var points = [40, 100, 1, 5, 25, 10];points.sort(function(a, b) { return b - a;});// now points[0] contains the highest value// and points[points.length-1] contains the lowest value
If you only want to find the top (or lowest) value, sorting an entire array is wasteful.
Using Math.max() on an Array: To determine the highest number in an array, use Math.max.apply:
JavaScript Code:
function myArrayMax(arr) { return Math.max.apply(null, arr);}Math.max.apply(null, [1, 2, 3]) is equivalent to Math.max(1, 2, 3).
Using Math.min() on an Array: To determine the lowest value in an array, use Math.min.apply:
JavaScript Code:
function myArrayMin(arr) { return Math.min.apply(null, arr);}
Math.min.apply(null, [1, 2, 3]) is equivalent to Math.min(1, 2, 3).
My Min / Max JavaScript Methods
Using a "home-made" approach is the quickest solution. This function iterates across an array, comparing each value to the highest value found:
JavaScript Code: (Find Max)
function myArrayMax(arr) { var len = arr.length; var max = -Infinity; while (len--) { if (arr[len] > max) { max = arr[len]; } } return max;}
This function iterates across an array, comparing each value to the lowest value found:
JavaScript Code: (Find Min)
function myArrayMin(arr) { var len = arr.length; var min = Infinity; while (len--) { if (arr[len] < min) { min = arr[len]; } } return min;}
Sorting Object Arrays: Objects are frequently found in JavaScript arrays
JavaScript Code:
var cars = [ { type: 'Volvo', year: 2016 }, { type: 'Saab', year: 2001 }, { type: 'BMW', year: 2010 },];
The sort() method can be used to sort an array even if its properties are of different data types. To compare the property values, write a compare function like follows:
JavaScript Code:
cars.sort(function(a, b) { return a.year - b.year;});
It's a little trickier to compare string properties:
JavaScript Code:
cars.sort(function(a, b) { var x = a.type.toLowerCase(); var y = b.type.toLowerCase(); if (x < y) { return -1; } if (x > y) { return 1; } return 0;});
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts