Tutorial: Javascript Number Methods
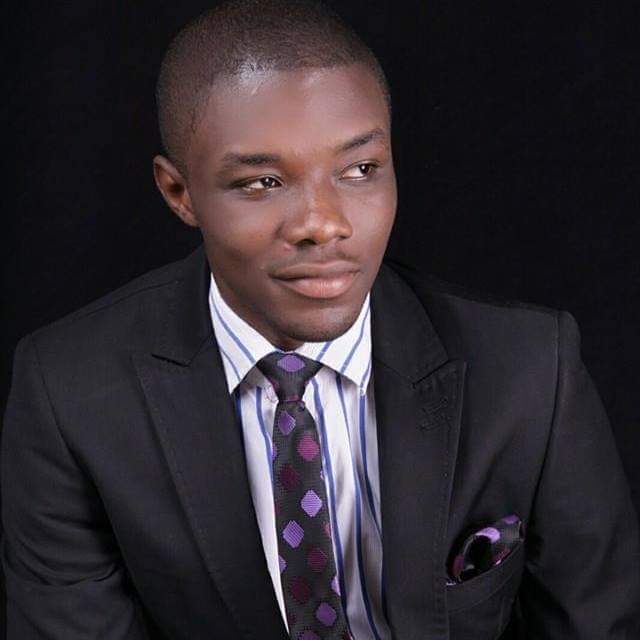
By Emmanuel Chinonso
Web Developer
JavaScript Number Methods
You can use number methods to work with numbers.
Number Methods and Properties
Property and method cannot be assigned to primitive values (such as 3.14 or 2014). (because they are not objects). However, primitive values can use JavaScript methods and properties because JavaScript interprets primitive values as objects when executing methods and properties.
The toString() Method The toString() method returns a string representation of an integer. Any sort of number can be utilized with any number procedure (literals, variables, or expressions).
JavaScript Code:
var x = 123;x.toString(); // returns 123 from variable x(123).toString(); // returns 123 from literal 123(100 + 23).toString(); // returns 123 from expression 100 + 23.
The toExponential() Method
toExponential() provides a string containing a number that has been rounded and expressed in exponential notation. The number of characters after the decimal point is defined by a parameter. The parameter is not required. JavaScript will not round the number if you don't specify it.
JavaScript Code:
var x = 9.656;x.toExponential(2); // returns 9.66e+0x.toExponential(4); // returns 9.6560e+0x.toExponential(6); // returns 9.656000e+0.
The valueOf() Method
valueOf() returns a number in its original form. A number can be either a primitive value (typeof = number) or an object (typeof = object) in JavaScript. In JavaScript, the valueOf() function is used to convert Number objects to primitive values. It's pointless to utilize it in your code.
JavaScript Code:
var x = 123;x.valueOf(); // returns 123 from variable x(123).valueOf(); // returns 123 from literal 123(100 + 23).valueOf(); // returns 123 from expression 100 + 23.
Converting Variables to Numbers
To convert variables to numbers, you can use one of three JavaScript methods:
- The parseInt() method
- The Number() method
- The method parseFloat()
These methods are global JavaScript methods, not numerical methods.
Global JavaScript Methods
All JavaScript data types can be utilized with global methods in JavaScript. When working with numbers, these are the most useful techniques:
Method | Description |
---|---|
Number() | Returns a number, converted from its argument. |
--- | --- |
parseFloat() | Parses its argument and returns a floating point number |
--- | --- |
parseInt() | Parses its argument and returns an integer |
The Number() Method; To convert JavaScript variables to numbers, use Number(). If the number cannot be converted, the result is NaN (Not a Number).
JavaScript Code:
Number(true); // returns 1Number(false); // returns 0Number('10'); // returns 10Number(' 10'); // returns 10Number('10 '); // returns 10Number(' 10 '); // returns 10Number('10.33'); // returns 10.33Number('10,33'); // returns NaNNumber('10 33'); // returns NaNNumber('John'); // returns NaN.
The Number() Method Used on Dates: A date can also be converted to a number using Number():
JavaScript Code:
Number(new Date('2017-09-30')); // returns 1506729600000
Since 1.1.1970, the Number() method returns the number of milliseconds.
The parseInt() Method: parseInt() returns a whole number after parsing a string. There are no restrictions on the number of spaces available. The first number is the only one that is returned:
JavaScript Code:
parseInt('10'); // returns 10parseInt('10.33'); // returns 10parseInt('10 20 30'); // returns 10parseInt('10 years'); // returns 10parseInt('years 10'); // returns NaN
If the number cannot be translated, the result is NaN (Not a Number).
The parseFloat() Method: parseFloat() returns a number after parsing a string. There are no restrictions on the number of spaces available. The first number is the only one that is returned:
JavaScript Code:
parseFloat('10'); // returns 10parseFloat('10.33'); // returns 10.33parseFloat('10 20 30'); // returns 10parseFloat('10 years'); // returns 10parseFloat('years 10'); // returns NaN.
Number Properties
Property | Description |
---|---|
MAX_VALUE | Returns the largest number possible in JavaScript |
--- | --- |
MIN_VALUE | Returns the smallest number possible in JavaScript |
--- | --- |
POSITIVE_INFINITY | Represents infinity (returned on overflow) |
--- | --- |
NEGATIVE_INFINITY | Represents negative infinity (returned on overflow) |
--- | --- |
NaN | Represents a "Not-a-Number" value. |
Variables Cannot Have Number Properties Number attributes are part of the Number number object wrapper in JavaScript. Only Number can be used to access these properties. MAX VALUE. I'm going to use myNumber. MAX VALUE will return undefined if myNumber is a variable, expression, or value:
JavaScript Code:
var x = 6;var y = x.MAX_VALUE; // y becomes undefined.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts