Tutorial: Javascript Boolean
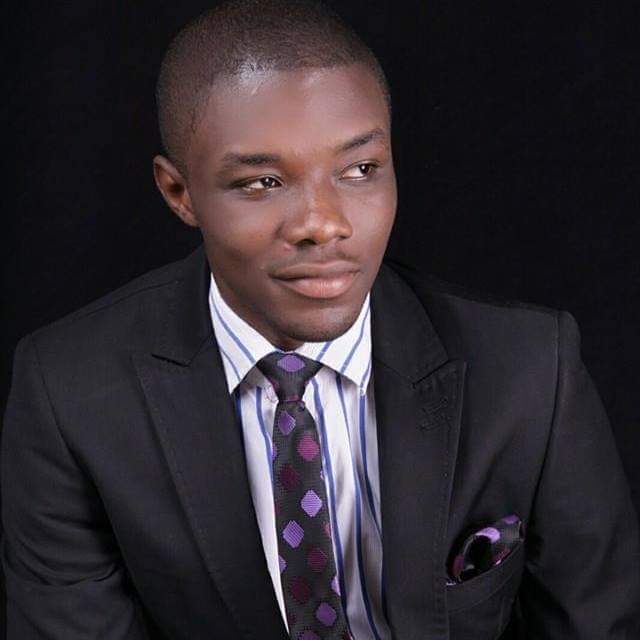
By Emmanuel Chinonso
Web Developer
JavaScript Booleans
True or false are the two values that a JavaScript Boolean represents.
Boolean Values
In programming, you'll frequently require a data type that can only have one of two values, like
- YES / NO
- ON / OFF
- TRUE / FALSE
JavaScript offers a Boolean data type for this. It can only take the true or false values.
The Boolean() Function: To determine whether an or a variable is true, use the Boolean() function:
JavaScript Code:
Boolean(10 > 9); // returns true
Or, to make things more simpler:
JavaScript Code:
10 > 9; // also returns true10 > 9; // also returns true
Comparisons and Conditions
Comparison Operators: In logical expressions, comparison operators are used to assess if variables or values are equal or different. The table below explains the comparison operators given x = 5:
Operator | Description | Comparing | Return |
---|---|---|---|
== | equal to | x == 8 | false |
------- | ------------ | ---------- | ------- |
== | equal to | x == "5" | true |
------- | ------------ | ---------- | ------- |
== | equal to | x == "5" | true |
------- | ------------ | ---------- | ------- |
=== | equal value and equal type | x === 5 | true |
------- | ------------ | ---------- | ------- |
=== | equal value and equal type | x === "5" | false |
------- | ------------ | ---------- | ------- |
!= | not equal | x != 8 | true |
------- | ------------ | ---------- | ------- |
!== | not equal value or not equal type | x !== 5 | false |
------- | ------------ | ---------- | ------- |
!== | not equal value or not equal type | x !== "5" | true |
------- | ------------ | ---------- | ------- |
!== | not equal value or not equal type | x !== 8 | true |
------- | ------------ | ---------- | ------- |
> | greater than | x > 8 | false |
------- | ------------ | ---------- | ------- |
< | less than | x < 8 | true |
------- | ------------ | ---------- | ------- |
>= | greater than or equal to | x >= 8 | false |
----------- | ------------ | ---------- | ------- |
<= | less than or equal to | x <= 8 | true |
Conditional statements are covered in detail in the chapter JS Conditions.
Here are some examples:
Operator | Description | JavaScript Code |
---|---|---|
== | equal to | if (day == "Monday") |
----------- | ------------ | ---------- |
> | greater than | if (salary > 9000) |
----------- | ------------ | ---------- |
< | less than | if (age < 18) |
All JavaScript comparisons and conditions are based on the Boolean value of an expression.
How Can it be Used
In conditional statements, comparison operators can be used to compare values and perform action based on the outcome.: if (age < 18) text = "Too young to buy alcohol";
Logical Operators The logic between variables or values is determined using logical operators. The table below explains the logical operators given x = 6 and y = 3:
Operator | Description | Example Try it |
---|---|---|
&& | and | (x < 10 && y > 1) is true |
----------- | ------------ | ---------- |
II | or | (x == 5 |
----------- | ------------ | ---------- |
! | nor | !(x == y) is true |
Conditional (Ternary) Operator: A conditional operator in JavaScript assigns a value to a variable based on a set of criteria.
Syntax
variablename = (condition) ? value1:value2
JavaScript Code:
var voteable = age < 18 ? 'Too young' : 'Old enough';
The value of voteable will be "Too young" if the variable age is less than 18, and "Old enough" if the variable age is greater than 18.
Comparing Different Types
When multiple types of data are compared, surprising results can occur. When comparing a string to a number, JavaScript converts the string to a number before comparing the two. The value of an empty string is 0. When a string is not numeric, it translates to NaN, which is always false.
Case | Value |
---|---|
2 < 12 | true |
------- | ------ |
2 < "12" | true |
------- | ------ |
2 < "John" | false |
------- | ------ |
2 > "John" | false |
------- | ------ |
2 == "John" | false |
------- | ------ |
"2" < "12" | false |
------- | ------ |
"2" > "12" | true |
------- | ------ |
"2" == "12" | false |
Because 1 is less than 2 (alphabetically), "2" will be greater than "12" when comparing two strings. Variables should be changed to the correct type before comparison to provide a good result:
age = Number(age);if (isNaN(age)) { voteable = 'Input is not a number';} else { voteable = age < 18 ? 'Too young' : 'Old enough';}
JavaScript Booleans are a powerful data type in JavaScript. They are used in conditionals, loops, functions, comparison operations, and more. Understanding how to use booleans correctly is essential to programming in JavaScript.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts