Tutorial: Javascript Dom Methods
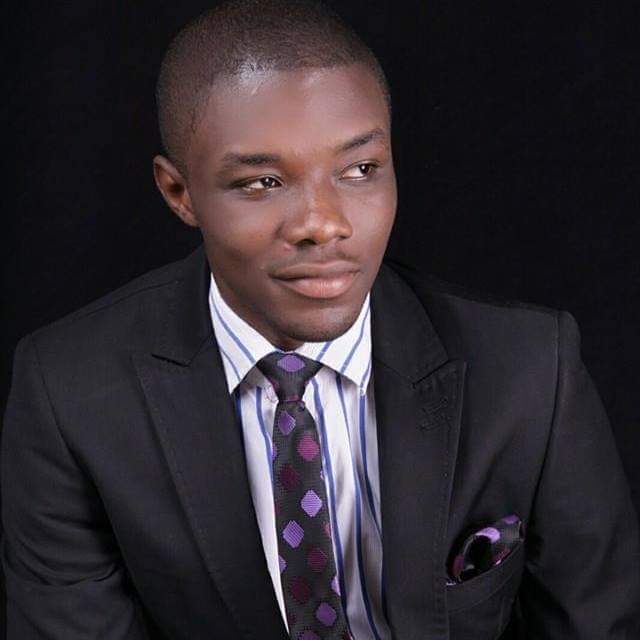
By Emmanuel Chinonso
Web Developer
JavaScript HTML DOM Methods
The DOM methods in HTML are actions that you can take (on HTML Elements). The values (of HTML Elements) that you can set or update are known as HTML DOM properties.
The DOM Programming Interface
JavaScript may access the HTML Document Object Model (DOM) (and with other programming languages). All HTML elements are defined as objects in the DOM. Each object's properties and methods make up the programming interface. A property is a value that may be obtained or changed (like changing the content of an HTML element). A method is an action that you can carry out (like add or deleting an HTML element).
The following code changes the content (the innerHTML) of the <p>
element with id="demo":
JavaScript Code:
<html> <body> <p id="demo"></p> <script>document.getElementById("demo").innerHTML = "Hello World!";</script> </body></html>
In the code above, getElementById is a method, while innerHTML is a property.
The getElementById Method
The most frequent method of accessing an HTML element is to use the element's id. The getElementById method in the code above used id="demo" to locate the element.
The innerHTML Property
The innerHTML property is the simplest way to access the content of an element. The innerHTML property can be used to access or replace HTML element content. Any HTML element, including <html>
and <body>
, can be retrieved or changed using the innerHTML property.
JavaScript HTML DOM Document
All other objects on your web page are owned by the HTML DOM document object. Your web page is represented by the document object. When you wish to get to any element on an HTML page, you must first get to the document object. Here are some examples of how you can read and alter HTML using the document object.
Finding HTML Elements
Method | Description |
---|---|
document.getElementById(id) | Find an element by element id |
------------------------------- | ---------------- |
document.getElementsByTagName(name) | Find elements by tag name |
------------------------------- | ---------------- |
document.getElementsByClassName(name) | Find elements by class name |
Changing HTML Elements
Property | Description |
---|---|
element.innerHTML = new html content | Change the inner HTML of an element |
------------------------------- | ---------------- |
element.attribute = new value Change | the attribute value of an HTML element |
------------------------------- | ---------------- |
element.style.property = new style | Change the style of an HTML element |
Method | Description |
---|---|
element.setAttribute(attribute, value) | Change the attribute value of an HTML element |
Adding and Deleting Elements
Method Description |
---|
document.createElement(element) |
------------------------------- |
document.removeChild(element) |
------------------------------- |
document.appendChild(element) |
------------------------------- |
document.replaceChild(new, old) |
------------------------------- |
document.write(text) |
JavaScript HTML DOM Elements: This page explains how to locate and use HTML components in an HTML document.
JavaScript is frequently used to manipulate HTML elements.
To accomplish so, you must first locate the elements. There are numerous options for doing so:
- Identifying HTML elements based on their id
- Identifying HTML elements by their tag names
- Locating HTML elements based on their class names
- CSS selectors for locating HTML elements
- Using HTML object collections to find HTML elements
- Locating an HTML Element based on its Id
The element id is the simplest way to locate an HTML element in the DOM. This code locates the element with the id="intro" attribute:
JavaScript Code:
var myElement = document.getElementById('intro');
The method will return the element as an object if the element is discovered (in myElement).MyElement will contain null if the element is not found.
Finding HTML Elements by Tag Name:
All <p>
elements are found using this code
JavaScript Code:
var x = document.getElementsByTagName('p');
This code locates the element with the id="main" and then all <p>
elements within it:
JavaScript Code:
var x = document.getElementById('main');var y = x.getElementsByTagName('p');
Finding HTML Elements by Class Name: Use getElementsByClassName to locate all HTML elements with the same class name (). This code generates a list of all elements that have the class="intro" attribute.
JavaScript Code:
var x = document.getElementsByClassName('intro');
In Internet Explorer 8 and previous versions, searching for elements by class name does not work.
Finding HTML Elements by CSS Selectors:
Use the querySelectorAll() method to locate all HTML elements that match a specific CSS selector (id, class names, types, attributes, attribute values, and so on). This code generates a list of all <p>
elements that have the class="intro" attribute..
JavaScript Code:
var x = document.querySelectorAll('p.intro');
In Internet Explorer 8 and previous versions, the querySelectorAll() method does not operate. HTML Elements Can Be Found Using HTML Object Collections This example searches the forms collection for the form element with the id="frm1" and displays all of its values:
JavaScript Code:
var x = document.forms['frm1'];var text = '';var i;for (i = 0; i < x.length; i++) { text += x.elements[i].value + '<br>';}document.getElementById('demo').innerHTML = text;
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts