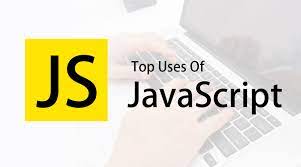
Tutorial: Javascript Classes
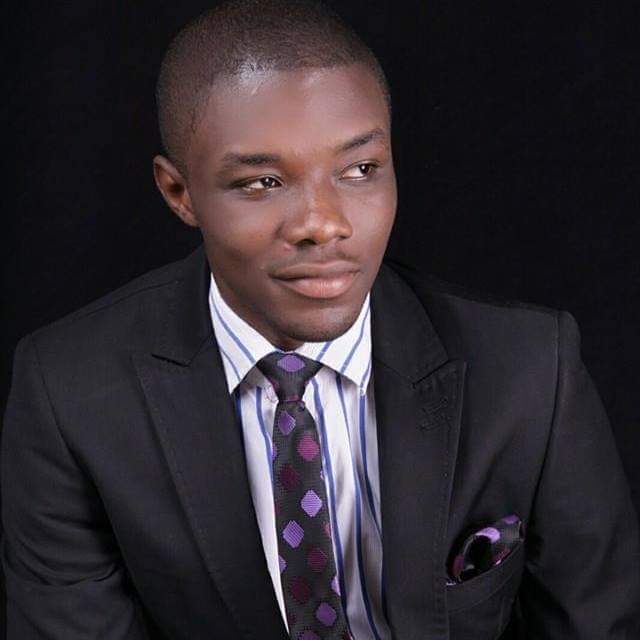
By Emmanuel Chinonso
Web Developer
JavaScript Classes
JavaScript Classes were introduced in ECMAScript 2015, often known as ES6. JavaScript Classes are JavaScript Object templates.
JavaScript Class Syntax
Use the class keyword to create a new class. Always include a constructor() method:
Syntax
class ClassName { constructor() { ... }}
JavaScript Code:
class Car { constructor(name, year) { this.name = name; this.year = year; }}
The code above generates a "Car" class. "Name" and "Year" are the two initial properties of the class. A JavaScript class isn't the same thing as an object. It is a JavaScript object template.
Using a Class: When you have a class, you may utilize it to make objects like these:
JavaScript Code:
let myCar1 = new Car('Ford', 2014);let myCar2 = new Car('Audi', 2019);
The Car class is used to construct two Car objects in the code above. When a new object is formed, the constructor method is automatically invoked.
The Constructor Method
The constructor method is unique:
- It must be named exactly "constructor"
- It is called automatically when a new object is created
- It is used to set up object properties
If you don't declare a constructor method, JavaScript creates one for you.
Class Methods
The same syntax applies to class methods as it does to object methods. To make a class, use the keyword class. A constructor() method should always be included. Then add as many methods as you want.
Syntax
class ClassName { constructor() { ... } method_1() { ... } method_2() { ... } method_3() { ... }}
Make a class method called "age" that returns the age of the car:
JavaScript Code:
class Car { constructor(name, year) { this.name = name; this.year = year; } age() { let date = new Date(); return date.getFullYear() - this.year; }}let myCar = new Car('Ford', 2014);document.getElementById('demo').innerHTML = 'My car is ' + myCar.age() + ' years old.';
You can send parameters to Class methods:
JavaScript Code:
class Car { constructor(name, year) { this.name = name; this.year = year; } age(x) { return x - this.year; }}let date = new Date();let year = date.getFullYear();let myCar = new Car('Ford', 2014);document.getElementById('demo').innerHTML = 'My car is ' + myCar.age(year) + ' years old.';
Class Inheritance
Use the extends keyword to construct a class inheritance. When a class is formed using class inheritance, it inherits all of the methods from the parent class: Create a "Model" class that inherits the methods from the "Car" class:
JavaScript Code:
class Car { constructor(brand) { this.carname = brand; } present() { return 'I have a ' + this.carname; }}class Model extends Car { constructor(brand, mod) { super(brand); this.model = mod; } show() { return this.present() + ', it is a ' + this.model; }}let myCar = new Model('Ford', 'Mustang');document.getElementById('demo').innerHTML = myCar.show();
The parent class is referred to by the super() function. We invoke the parent's constructor method and gain access to the parent's properties and methods by calling the super() function in the constructor method. Inheritance is important for code reuse since it allows you to reuse attributes and functions from another class when creating a new one.
Getters and Setters You can also use getters and setters with classes. Using getters and setters for your properties might be beneficial, especially if you want to do anything unique with the value before returning it or setting it. Use the get and set keywords to add getters and setters to the class. For the "carname" property, make a getter and a setter
JavaScript Code:
class Car { constructor(brand) { this.carname = brand; } get cnam() { return this.carname; } set cnam(x) { this.carname = x; }}let myCar = new Car('Ford');document.getElementById('demo').innerHTML = myCar.cnam;
When you want to get the property value from a getter that is a method, you don't use parentheses. The getter/setter method's name cannot be the same as the property's name, in this example carname. To distinguish the getter/setter from the actual property, many programmers use the underscore character before the property name: To distinguish the getter/setter from the actual property, use the underscore character:
JavaScript Code:
class Car { constructor(brand) { this._carname = brand; } get carname() { return this._carname; } set carname(x) { this._carname = x; }}let myCar = new Car('Ford');document.getElementById('demo').innerHTML = myCar.carname;
Use the same syntax as when setting a property value, but without the parentheses:Use a setter to change the carname to "Volvo":
JavaScript Code:
class Car { constructor(brand) { this._carname = brand; } get carname() { return this._carname; } set carname(x) { this._carname = x; }}
let myCar = new Car('Ford');myCar.carname = 'Volvo';document.getElementById('demo').innerHTML = myCar.carname;
Hoisting Class declarations, unlike functions and other JavaScript declarations, are not hoisted. That is, before you can use a class, you must first declare it:
JavaScript Code:
//You cannot use the class yet.//myCar = new Car("Ford")//This would raise an error.class Car { constructor(brand) { this.carname = brand; }}//Now you can use the class:let myCar = new Car('Ford');
When you try to use additional declarations, such as functions, before they are declared, you will not get an error because the default behavior of JavaScript declarations is hoisting (moving the declaration to the top). The class's static methods are declared on the class's level. A static method can only be called on an object class, not on an individual object.
JavaScript Code:
class Car { constructor(name) { this.name = name; } static hello() { return 'Hello!!'; }}let myCar = new Car('Ford');// You can calll 'hello()' on the Car Class:document.getElementById('demo').innerHTML = Car.hello();
// But NOT on a Car Object:// document.getElementById("demo").innerHTML = myCar.hello();// this will raise an error.
You can pass the myCar object as an argument to the static method if you want to use it:
JavaScript Code:
class Car { constructor(name) { this.name = name; } static hello(x) { return 'Hello ' + x.name; }}let myCar = new Car('Ford');document.getElementById('demo').innerHTML = Car.hello(myCar);
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
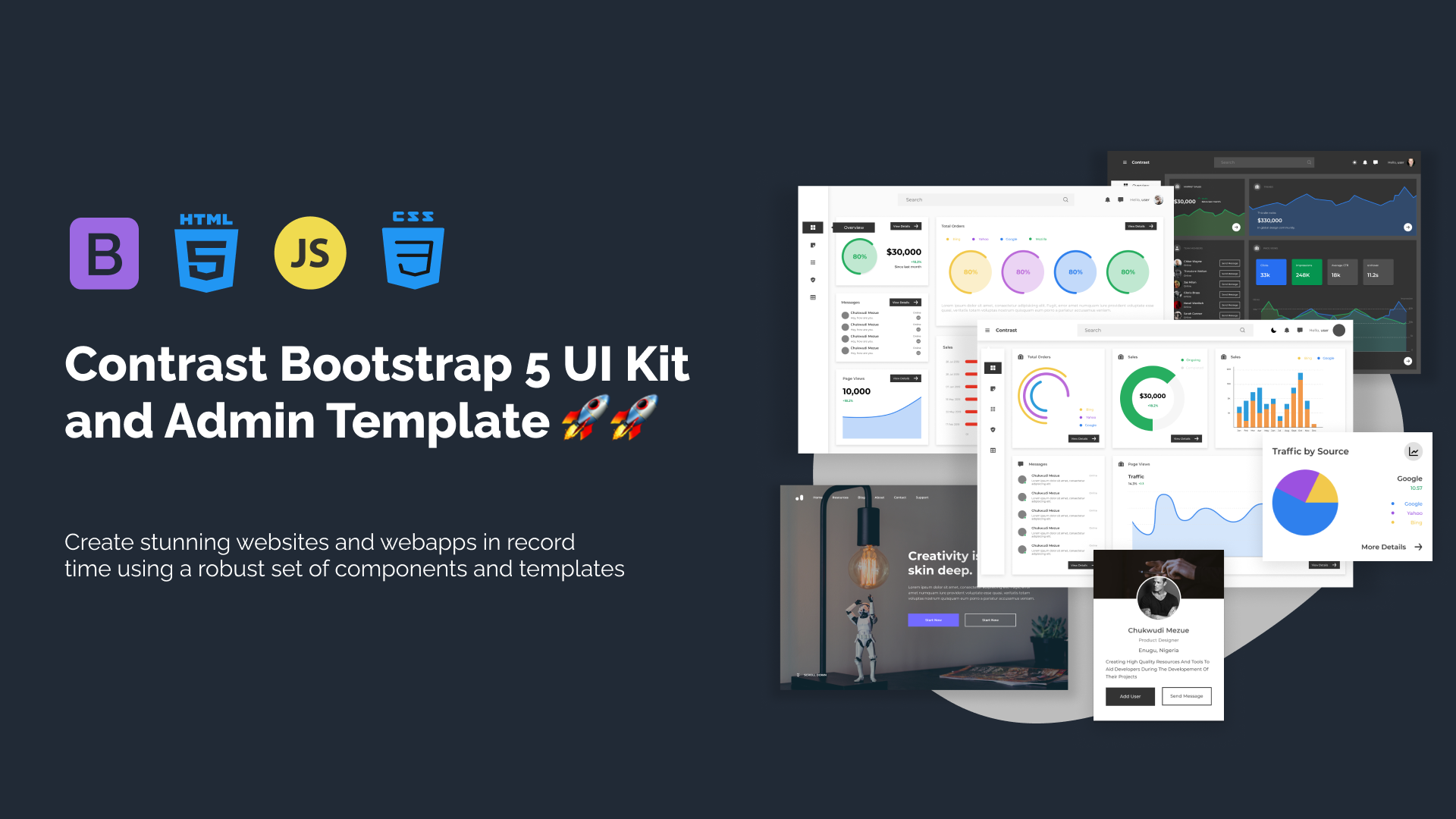
Related Posts
Comments
...