Tutorial: Javascript Control Flow
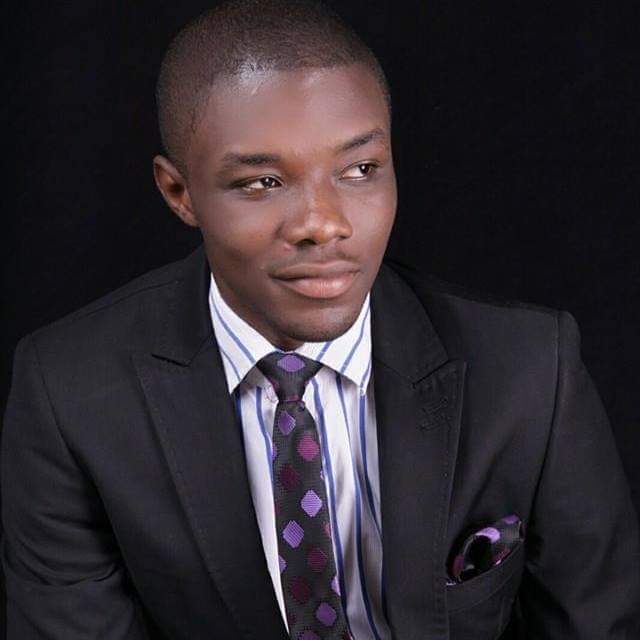
By Emmanuel Chinonso
Web Developer
Javascript Control Flow
Conditional statements are used to perform a variety of actions in response to a variety of circumstances.
Conditional Statements
When writing code, it's common to wish to perform different actions based on different decisions. To do this, you can utilize conditional statements in your code. The conditional statements below are accessible in
- If a specific condition is true, use if to specify a block of code to be executed.
- If the same condition is false, use else to specify a block of code to be executed.
- If the first condition is false, use else if to define a new condition to test.
- Use the switch to define a large number of different code blocks to run.
The if Statement
- If a condition is true, use the if statement to specify a block of JavaScript code to run. Syntax:
if (condition) { // block of code to be executed if the condition is true}
It's worth noting that 'if' is written in lowercase characters. A JavaScript problem will occur if you use uppercase letters (If or IF). If the time is less than 18:00, greet them with a "Good day" greeting:
JavaScript Code:
if (hour < 18) { greeting = 'Good day';}
The greeting will have the following result: Good day. The else Statement If the condition is false, use the else statement to specify a block of code to run.
if (condition) { // block of code to be executed if the condition is true} else { // block of code to be executed if the condition is false}
Create a "Good day" greeting if the hour is less than 18, else "Good evening":
JavaScript Code:
if (hour < 18) { greeting = 'Good day';} else { greeting = 'Good evening';}
The following is the outcome of greeting:
Good day The else if Statemen If the first condition is false, use the else if statement to provide a new condition.
Syntax
if (condition1) { // block of code to be executed if condition1 is true} else if (condition2) { // block of code to be executed if the condition1 is false and condition2 is true} else { // block of code to be executed if the condition1 is false and condition2 is false}
Produce a "Good morning" greeting if the time is less than 10:00; if not, but the time is less than 20:00, create a "Good day" greeting; otherwise, a "Good evening" greeting:
JavaScript Code:
if (time < 10) { greeting = 'Good morning';} else if (time < 20) { greeting = 'Good day';} else { greeting = 'Good evening';}
The greeting will have the following result: Good day.
JavaScript Switch Statement
The switch statement is used to conduct several actions depending on certain criteria. The switch statement is used to select one of many code blocks to run.
Syntax
switch (expression) { case x: // code block break; case y: // code block break; default: // code block}
This is how it works: Only one time is the switch expression evaluated. The expression's value is compared against the values in each circumstance. If a match is found, the relevant code block is run. If no match is found, the default code block is run. The weekday is returned as a number between 0 and 6 by the getDay() method. (Sunday=0, Monday=1, Tuesday=2, and so forth.) This code calculates the weekday name using the weekday number:
JavaScript Code:
switch (new Date().getDay()) { case 0: day = 'Sunday'; break; case 1: day = 'Monday'; break; case 2: day = 'Tuesday'; break; case 3: day = 'Wednesday'; break; case 4: day = 'Thursday'; break; case 5: day = 'Friday'; break; case 6: day = 'Saturday';}
The result of day will be: Saturday
The break Keyword
JavaScript exits the switch block when it reaches the break keyword. This will bring the execution of the switch block to a halt. The last case in a switch block does not need to be broken. In any case, the block breaks (terminates) there. If you don't use the break statement, even if the evaluation doesn't match the case, the next case will be processed.
The default Keyword
If there is no case match, the default keyword indicates the code to run: The weekday is returned as a number between 0 and 6 by the getDay() method. Write a default message if today is not Saturday (6) nor Sunday (0), write a default message:
JavaScript Code:
switch (new Date().getDay()) { case 6: text = 'Today is Saturday'; break; case 0: text = 'Today is Sunday'; break; default: text = 'Looking forward to the Weekend';}
The result of text will be: Today is Saturday
In a switch block, the default case does not have to be the last case
JavaScript Code:
switch (new Date().getDay()) { default: text = 'Looking forward to the Weekend'; break; case 6: text = 'Today is Saturday'; break; case 0: text = 'Today is Sunday';}
Remember to terminate the default case with a break if it isn't the last case in the switch block.
Common Code Blocks
Occasionally, you'll want to utilize the same code for multiple switch scenarios. Cases 4 and 5 share the same code block, while 0 and 6 share a different code block:
JavaScript Code:
switch (new Date().getDay()) { case 4: case 5: text = 'Soon it is Weekend'; break; case 0: case 6: text = 'It is Weekend'; break; default: text = 'Looking forward to the Weekend';}
Switching Details
If more than one case fits a case value, the first one is chosen. If no cases are identified that match, the program defaults to the default label. If no default label can be discovered, the program moves on to the statement(s) after the switch.
Strict Comparison
A strict comparison (===) is used in switch scenarios. In order for the values to match, they must be of the same type. Only when the operands are of the same type can a rigorous comparison be made. There won't be a match for x in this code:
JavaScript Code:
var x = '0';switch (x) { case 0: text = 'Off'; break; case 1: text = 'On'; break; default: text = 'No value found';}
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts