Tutorial: Javascript Arrays
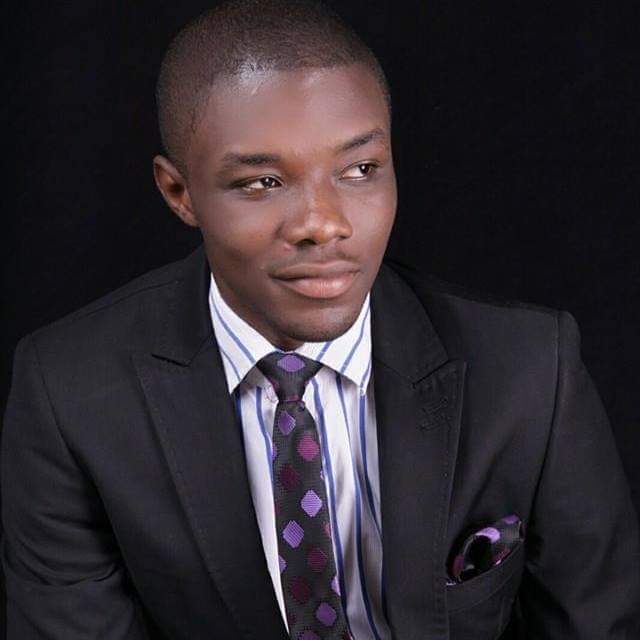
By Emmanuel Chinonso
Web Developer
JavaScript Arrays
Arrays in JavaScript are used to store several values in a single variable.
JavaScript Code:
var cars = ['Saab', 'Volvo', 'BMW'];
What is an Array?
An array is a type of variable that may store several values at the same time. If you have a list of objects (for example, a list of automobile names), storing the vehicles in single variables could look like this:
- var car1 = "Saab";
- var car2 = "Volvo";
- var car3 = "BMW";
But what if you want to go through the automobiles one by one and find a certain one? What if you had 300 vehicles rather than three? An array is the solution! A single name can be used to store many values in an array, and the data can be accessed by referring to an index number.
Creating an Array:
The simplest approach to generate a JavaScript Array is to use an array literal.
Syntax:
var array_name = [item1, item2, ...];
JavaScript Code:
var cars = ['Saab', 'Volvo', 'BMW'];
It is not necessary to use spaces or line breaks. A declaration can consist of numerous lines:
JavaScript Code:
var cars = ['Saab', 'Volvo', 'BMW'];
Using the JavaScript Keyword new: The following code also creates an Array, and assigns values to it:
JavaScript Code:
var cars = new Array('Saab', 'Volvo', 'BMW');
The previous two instances do the same thing. It is not necessary to utilize new Array (). Use the first one(the array literal method) for simplicity, readability, and execution speed.
Access the Elements of an Array
The index number is used to access an array element.
The value of the first element in cars is accessed by this statement.
The indexes of arrays begin with 0.
The first element is [0]. The second element is [1].
var name = cars[0];
JavaScript Code:
var cars = ['Saab', 'Volvo', 'BMW'];document.getElementById('demo').innerHTML = cars[0];
Changing an Array Element:
The value of the first element in vehicles is changed by this statement: cars[0] = "Opel"; JavaScript Code:
var cars = ['Saab', 'Volvo', 'BMW'];cars[0] = 'Opel';document.getElementById('demo').innerHTML = cars[0];
Access the Full Array: The complete array can be obtained in JavaScript by referring to the array name:
JavaScript Code:
var cars = ['Saab', 'Volvo', 'BMW'];document.getElementById('demo').innerHTML = cars;
Arrays are Objects
Arrays are a type of object. For arrays, the typeof operation in JavaScript returns "object." However, JavaScript arrays are better described as arrays. Arrays employ numbers to access their "elements." In this case, person[0] returns John:
Array:
var person = ['John', 'Doe', 46];
Objects use names to access their "members." In this case, person.firstName returns John:
Object:
var person = { firstName: 'John', lastName: 'Doe', age: 46 };
Array Elements Can Be Objects Objects can be used as variables in JavaScript. Arrays are specialized types of objects. As a result, you can have variables of different types in the same Array. An array can contain objects. An Array can contain functions. Arrays can contain arrays:
myArray[0] = Date.now;myArray[1] = myFunction;myArray[2] = myCars;
Array Properties and Methods: The built-in array attributes and methods are the real strength of JavaScript arrays:
JavaScript Code:
var x = cars.length; // The length property returns the number of elementsvar y = cars.sort(); // The sort() method sorts arrays
The length Property
The length property of an array returns the array's length (the number of array elements). The length property is always one more than the array index with the highest value.
JavaScript Code:
var fruits = ['Banana', 'Orange', 'Apple', 'Mango'];fruits.length; // the length of fruits is 4
Accessing the First Array Element
JavaScript Code:
fruits = ['Banana', 'Orange', 'Apple', 'Mango'];var first = fruits[0];
Accessing the Last Array Element JavaScript Code:
fruits = ['Banana', 'Orange', 'Apple', 'Mango'];var last = fruits[fruits.length - 1];
Looping Array Elements: The safest way to loop through an array, is using a for loop:
JavaScript Code:
var fruits, text, fLen, i;fruits = ['Banana', 'Orange', 'Apple', 'Mango'];fLen = fruits.length;
text = '<ul>';for (i = 0; i < fLen; i++) { text += '<li>' + fruits[i] + '</li>';}text += '</ul>';
You can also use the Array.forEach() function:
JavaScript Code:
var fruits, text;fruits = ['Banana', 'Orange', 'Apple', 'Mango'];text = '<ul>';fruits.forEach(myFunction);text += '</ul>';
function myFunction(value) { text += '<li>' + value + '</li>';}
Adding Array Elements: The push() method is the simplest way to add a new element to an array:
JavaScript Code:
var fruits = ['Banana', 'Orange', 'Apple', 'Mango'];fruits.push('Lemon'); // adds a new element (Lemon) to fruits
The length property can also be used to add new elements to an array.
Adding elements with high indices to an array can result in undefined "holes": JavaScript Code:
var fruits = ['Banana', 'Orange', 'Apple', 'Mango'];fruits[fruits.length] = 'Lemon'; // adds a new element (Lemon) to fruits
JavaScript Code:
var fruits = ['Banana', 'Orange', 'Apple', 'Mango'];fruits[6] = 'Lemon'; // adds a new element (Lemon) to fruits
Associative Arrays
Arrays with named indexes are supported by a wide range of programming languages. Associative arrays are arrays with named indexes (or hashes). Arrays with named indexes are not supported by JavaScript. Arrays in JavaScript always utilize numbered indexes. When using named indexes, JavaScript will redefine the array to a normal object.
Following that, several array methods and properties will yield inaccurate results
JavaScript Code:
var person = [];person[0] = 'John';person[1] = 'Doe';person[2] = 46;var x = person.length; // person.length will return 3var y = person[0]; // person[0] will return "John"
JavaScript Code:
var person = [];person['firstName'] = 'John';person['lastName'] = 'Doe';person['age'] = 46;var x = person.length; // person.length will return 0var y = person[0]; // person[0] will return undefined
The Difference Between Arrays and Objects
- Arrays in JavaScript employ numbered indexes.
- Objects in JavaScript make use of named indexes.
- Arrays are a type of object that has numbered indexes.
When to Use Arrays. When to use Objects.
Associative arrays are not supported by JavaScript. When you want the element names to be strings, you should use objects (text). When you want the element names to be numbers, you should use arrays
Avoid new Array() There is no need to utilize the built-in array constructor new Array in JavaScript ().
Instead, use [].
Both of these statements generate a new empty array named points:
var points = new Array(); // Badvar points = []; // Good
Both of these statements generate a new array of 6 numbers:
var points = new Array(40, 100, 1, 5, 25, 10); // Badvar points = [40, 100, 1, 5, 25, 10]; // Good
The new keyword only adds to the code's complexity. It can also have some unforeseen outcomes:
var points = new Array(40, 100); // Creates an array with two elements (40 and 100)
What if I take one of the elements out?
var points = new Array(40); // Creates an array with 40 undefined elements !!!!!.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts