How to create beautiful React Bootstrap 5 tabs
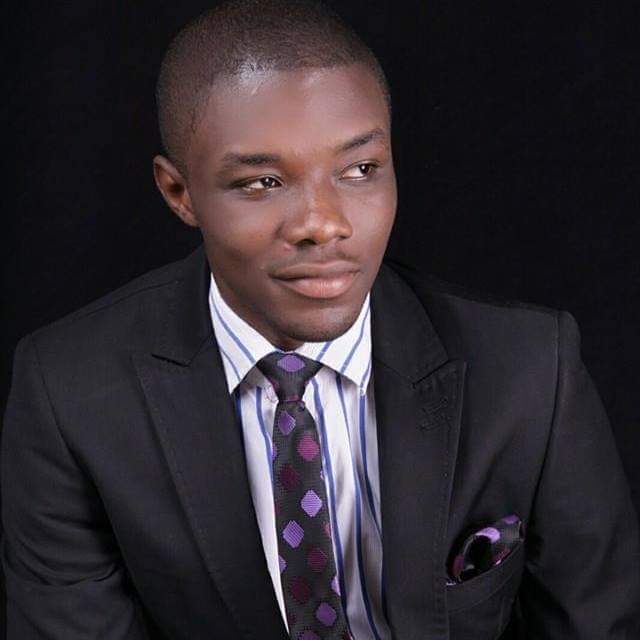
By Emmanuel Chinonso
Web Developer
React Boostrap 5 Tabs
React Bootstrap 5 Tabs are an essential component for creating organized and user-friendly interfaces in web applications. They allow you to separate content into different panes, with only one pane visible at a time, making it easier for users to navigate through various sections of your application without overwhelming them with information.
Table of Content
- Introduction
- Prerequisites
- Setup
- Installing CDBReact
- React Bootstrap 5 Tab Examples
- Difference Between Bootstrap Tabs and Pills
- Responsive Bootstrap Tab Menu
- Disabling Tabs in Bootstrap 5
- Conclusion
- Resources
Introduction
In this tutorial, we will guide you through the process of creating beautiful and functional tabs using the react-bootstrap
library known as Contrast (CDBReact). Whether you are a beginner or an experienced developer, this step-by-step guide will help you integrate React Bootstrap 5 Tabs into your project seamlessly. By the end of this tutorial, you will have a solid understanding of how to set up, customize, and use these tabs to enhance the navigation and visual appeal of your React applications.
Prerequisites
To follow along, you should have:
- Basic knowledge of JavaScript, React, and Bootstrap.
- Node.js and npm installed. Verify with
node -v
andnpm -v
. - Alternatively, you can use Yarn as a package manager.
Setup
First Check that you have the node installed. To do this, run the following command in your terminal.
node -v
This should show you the current version of the node you have installed on your machine. If you don’t have node.js installed, download it here. Installing node also installs npm on your PC.
Now that we have the node installed, we can start up our React project by going to the directory of our choice and running.
npx create-react-app tab-app
I named the project tab-app
but you can use whatever name of your choice.
Installing CDBReact-pro
Before we install our CDBReact we have to download the pro version first. Then, we can go ahead to install the react-bootstrap to build the tab component. It is advised to add the file to the root of the project by running.
npm install --save ./path-to-the-cdbreact-pro-tgz-file
Or using Yarn
yarn add ./path-to-the-cdbreact-pro-tgz-file
Our tab would be making use of the Navlink component from React router, so let us install it by running the command below.
Code:
npm install react-router-dom
Note : React Bootstrap Tabs are compatible with ReactRouter, meaning that <NavLink>
components inside can be used for one-page tabs and general routing solutions for the main navigation. Make sure to include the wrapping <BrowserRouter>
.
If you are using the navbar to provide a navigation bar, be sure to add appropriate role attributes to the <NavLinks>
(role="tab").
Now run npm start
to make sure that everything works well and there are no errors.
Before we proceed, let’s go ahead and wrap our app with the BrowserRouter component from react-router-dom as Navlinks can’t work outside it.
import './App.css';import Tab from './tab';import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"></div> </Router> );}
export default App;
React Bootstrap Tabs Examples
Create a file named tab.js
and import the necessary components:
import React from "react";import { CDBNav, CDBTabLink, CDBTabContent, CDBTabPane, CDBContainer } from "cdbreact";import { NavLink } from react-router-dom;
const Tab = () => { return ( <CDBContainer> <CDBContainer> <CDBNav className="nav-tabs mt-5"> <CDBTabLink link to="#" active={this.state.activeItem === '1'} onClick={this.toggle('1')} role="tab" > Label 1 </CDBTabLink>
<CDBTabLink link to="#" active={this.state.activeItem === '2'} onClick={this.toggle('2')} role="tab" > Label 2 </CDBTabLink> <CDBTabLink link to="#" active={this.state.activeItem === '3'} onClick={this.toggle('3')} role="tab" > Label 3 </CDBTabLink> </CDBNav> <CDBTabContent activeItem={this.state.activeItem}> <CDBTabPane tabId="1" role="tabpanel"> <p className="mt-2"> Lorem ipsum dolor sit amet, consectetur adipisicing elit. Nihil odit magnam minima, soluta doloribus reiciendis molestiae placeat unde eos molestias. Quisquam aperiam, pariatur. Tempora, placeat ratione porro voluptate odit minima. </p> </CDBTabPane> <CDBTabPane tabId="2" role="tabpanel"> <p className="mt-2"> Quisquam aperiam, pariatur. Tempora, placeat ratione porro voluptate odit minima. Lorem ipsum dolor sit amet, consectetur adipisicing elit. Nihil odit magnam minima, soluta doloribus reiciendis molestiae placeat unde eos molestias. </p> <p> Quisquam aperiam, pariatur. Tempora, placeat ratione porro voluptate odit minima. Lorem ipsum dolor sit amet, consectetur adipisicing elit. Nihil odit magnam minima, soluta doloribus reiciendis molestiae placeat unde eos molestias. </p> </CDBTabPane> <CDBTabPane tabId="3" role="tabpanel"> <p className="mt-2"> Quisquam aperiam, pariatur. Tempora, placeat ratione porro voluptate odit minima. Lorem ipsum dolor sit amet, consectetur adipisicing elit. Nihil odit magnam minima, soluta doloribus reiciendis molestiae placeat unde eos molestias. </p> </CDBTabPane> </CDBTabContent> </CDBContainer> </CDBContainer> );};export default Tab;
in the file above, we imported some CDBReact for the tab.
- CDBNav for the navigation of the web.
- CDBTabLink for links in the tab.
- CDBTabContent contains the main body of the tab.
- CDBTabPane contains the pane of the tab.
- CDBContainer wraps the entire components of the tab.
we used CDBNav
, CDBTabLink
, CDBTabContent
, CDBTabPane
, CDBContainer
to create the tab and add contents with some react-bootstrap styling.
Let us go ahead to import the created tab component into our app component
import './App.css';import Tab from './tab';import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"> <Tab /> </div> </Router> );}
export default App;
The tab component we created will look like the image below
Difference Between Bootstrap Tabs and Pills
Tabs and Pills are part of the tab component in Bootstrap that let you navigate through different content sections. The major feature difference is in how they look,
Tabs : another old way to navigate links, which are rectangular with typical tabbed interface meant for content directly linked that is relying again on wide patterns.
Pills: Pills are browsers site with a fetal interface on the menu or for good looking modern and flatolvency.
In the CDBNav component, you can simply change nav-tabs
to nav-pills
, and it will toggle between tabs with pills.
Responsive Bootstrap Tab Menu
Bootstrap 5 provides built-in support for responsive tab menus. To make your tabs responsive, ensure that your layout uses Bootstrap's grid system and responsive utility classes. For example, you can wrap your tabs in a container that adjusts based on screen size
Disabling Tabs in Bootstrap 5
To disable a tab in Bootstrap 5, you can add the disabled class to the CDBTabLink component. This will make the tab unclickable and visually indicate that it is disabled:
<CDBTabLink link to="#" active={activeItem === '1'} onClick={toggle('1')} role="tab"> Label 1</CDBTabLink><CDBTabLink link to="#" active={activeItem === '2'} onClick={toggle('2')} role="tab" className="disabled"> Label 2 (Disabled)</CDBTabLink><CDBTabLink link to="#" active={activeItem === '3'} onClick={toggle('3')} role="tab"> Label 3</CDBTabLink>
Conclusion
React Bootstrap 5 tabs are a powerful tool for organizing and presenting content in a user-friendly manner. By following the steps outlined in this article, you can create beautiful and functional tabs that enhance the navigation and visual appeal of your React applications. Experiment with customization options and design choices to create tabs that align with your application's style and purpose.
Resources
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts