How to create a beautiful React Bootstrap table.
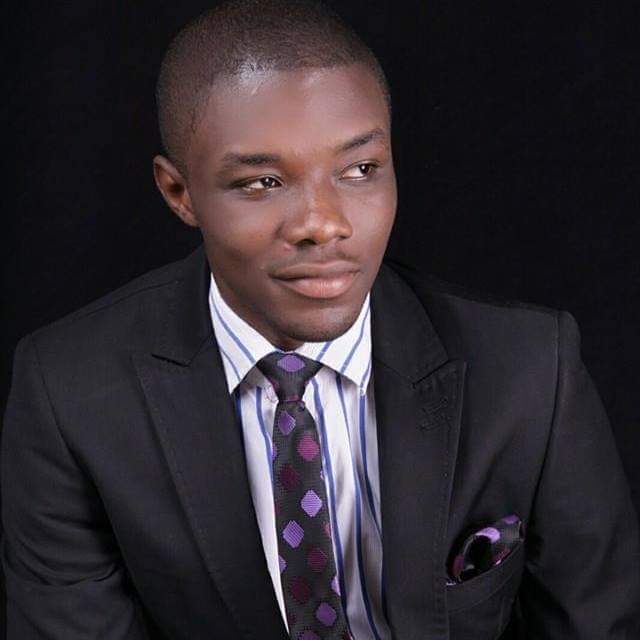
By Emmanuel Chinonso
Web Developer
React Bootstrap 5 Table
React Bootstrap 5 tables are components with basic table features. They let you aggregate a massive amount of data and present it in a clear and orderly way. These tables provide additional benefits like responsiveness and the possibility to manipulate the styles of the tables. You can enhance your tables by adding buttons, checkboxes, panels, and many other additional elements. You can also use advanced data table options like sort, search, or pagination. All these make the react Bootstrap table editable.
Table of Content
- Introduction
- Prerequisites
- Setup
- Installing CDBReact
- React Bootstrap 5 Table Examples
- Conclusion
- Resources
Introduction
Today, we’ll be creating a table in react using a react-bootstrap library known as Contrast. Contrast, also known as CDBReact is a react library which is an Elegant UI kit with full bootstrap support that has reusable components for building mobile-first, responsive websites and web apps.
Prerequisites
To follow this guide, you need:
- JavaScript Knowledge
- Basic React Knowledge
- Basic Bootstrap Knowledge
- Node.js and npm installed
Setup
First Check that you have the node installed. To do this, run the following command in your terminal.
node -v
This should show you the current version of the node you have installed on your machine. If you don’t have node.js installed, download it here. Installing node also installs npm on your PC.
Install React
we can start up our React project by going to the directory of our choice and running.
npx create-react-app table-app
I named the project table-app
but you can use any name of your choice.
Installing CDBReact
To Install CDBReact, run the following command on your terminal
npm install --save cdbreact
Or using Yarn
yarn add cdbreact
Note that we don’t need to install bootstrap or add it anywhere in our project as CDBReact does that for us upon installation.
React Bootstrap 5 Table Examples
Basic Table
Create a file named table.js
and import the necessary components:
import React from "react";import { CDBTable, CDBTableHeader, CDBTableBody, CDBContainer } from "cdbreact";
const Table = () => { return ( <CDBContainer> <CDBTable responsive> <CDBTableHeader> <tr> <th>#</th> <th>First</th> <th>Second</th> <th>Third</th> <th>Fourth</th> <th>Fifth</th> <th>Sixth</th> <th>Seventh</th> <th>Last</th> <th>Handle</th> </tr> </CDBTableHeader> <CDBTableBody> <tr> <td>1</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>@email</td> </tr> <tr> <td>2</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>@email</td> </tr> <tr> <td>3</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>Name</td> <td>@email</td> </tr> </CDBTableBody> </CDBTable> </CDBContainer> );};
export default Table;
In the code above, we imported the table components to help us create different tables. These components include:
CDBTable
is the table itselfCDBTableHeade
r, for creating table headers.CDBTableBody
, to create the body of the tableCDBContainer
, uses the entire component.
Now, we are going to render the component into our app.js
.
import './App.css';import Table from './table';import Reactdom from 'react-dom';
function App() { return ( <div className="App"> <Table /> </div> );}
Reactdom.render(<App />, document.getElementById('root'));
Our page will look like the image below
React Bootstrap 5 Editable Table
To create a React bootstrap editable table, you can use state to manage the table data and provide input fields for editing.
Note You require the CDBReact pro to build this Responsive React Bootstrap 5 editable table. You can check the step by step guild to install the CDBReact Pro.
import React from 'react';import { CDBEditableTable, CDBCard, CDBCardBody, CDBContainer } from 'cdbreact';
const EditableTable = () => { const columns = ['Fullname', 'Age', 'Company Name', 'City', 'Country'];
const data = [ ['Guerra Cortez', 45, 'Insectus', 'San Francisco', 'USA'], ['Elisa Gallagher', 31, 'Portica', 'London', 'United Kingdom'], ['Aurelia Vega', 30, 'Deepends', 'Madrid', 'Spain'], ['Guadalupe House', 26, 'Isotronic', 'Berlin', 'Germany'], ];
return ( <CDBContainer> <CDBCard> <CDBCardBody> <CDBEditableTable striped bordered data={data} columns={columns} /> </CDBCardBody> </CDBCard> </CDBContainer> );};
export default EditableTable;
In the code above, the components were used to build a card body and editable table, and some styles were added to the components to enable them to be more presentable.
The next step is to render the components in the app.js
.
import './App.css';import EditableTable from './Editabletable';
function App() { return ( <Router> <div className="App"> <EditableTable /> </div> </Router> );}
export default App;
The code above shows the editable table components in the root app, which allows the application to be presented on the web.
This is how the page will look now.
Conclusion
You have now created a beautiful and functional React Bootstrap 5 table using CDBReact. This guide covered creating a basic table, making it responsive, and adding editable fields. Whether you are a beginner or an experienced developer, this guide should help you integrate a table component into your React application with ease.
Resources
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts