Bootstrap 5 stepper-How to create a beautiful Bootstrap 5 stepper
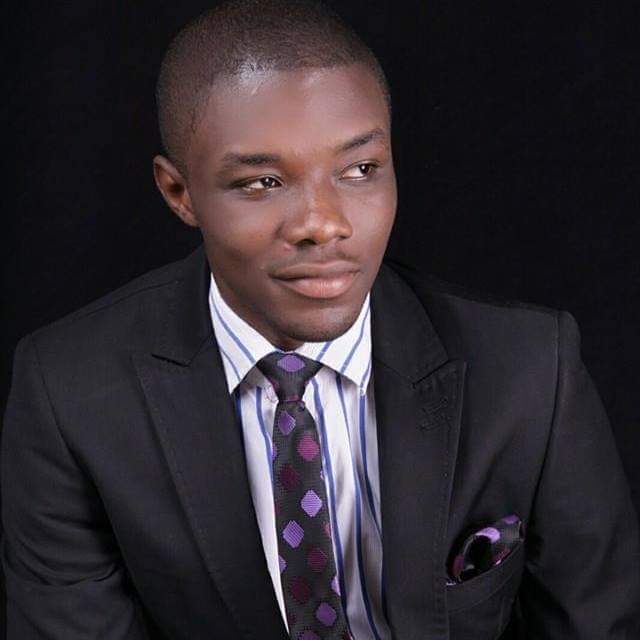
By Emmanuel Chinonso
Web Developer
React Bootstrap 5 Stepper?
A React Bootstrap 5 Stepper is a component that displays content as a process defined by user milestones. The following steps are separated and connected by buttons.This is an excellent solution for various registration forms, where you don't want to scare the user with loads of fields and questions. We can use the stepper to ease the entire registration process one data input at a time.
React Bootstrap 5 Stepper can be aligned vertically as well as horizontally.
Table of Contents
- Introduction
- Prerequisites
- Setup
- Installing CDBReact-Pro
- React Bootstrap 5 Stepper Example
- Conclusion
- Resources
Introduction
We will look at how to create this React Bootstrap 5 Stepper today using a react library known as Contrast or CDBReact.
CDBReact is a react library which is an Elegant UI kit with full bootstrap 5 support that has reusable components for building mobile-first, responsive websites and web apps.
To use Bootstrap 5 Stepper, you need to download the pro version here.
Prerequisites
- Basic React Knowledge
- JavaScript knowledge
- Basic Bootstrap knowledge
- NPM installed
Setup
First, you check if you have a node installed. To do this, run the following command in your terminal.
node - v;
This should show you the current version of the node you have installed on your machine. If you don’t have a node installed, download it here. Installing node also installs npm on your PC.
Installing React Project
Now that we have the node installed, we can start our React project by going to the directory of our choice and running.
npx create-react-app stepper-app
I named the project Stepper-app
, but you can use any name of your choice.
Installing CDBReact-pro
Before we install our CDBReact we have to download the pro version here first. Then, we can go ahead to install them in our project. It is advised to add the file to the root of the project by running:
npm install --save ./path-to-the-cdbreact-pro-tgz-file
Or using Yarn
yarn add ./path-to-the-cdbreact-pro-tgz-file
Note that we don’t need to install bootstrap or add it anywhere in our project, as CDBReact does that for us upon installation.
React Bootstrap 5 Stepper Example
We can now create a file named stepper.js
. This is where we are going to import the Stepper and other components. This is also where we will be writing our code.
The CDBReact stepper component must first be imported before using it.
import React, { useState } from 'react';import { CDBStepper, CDBStep, CDBInput, CDBBtn, CDBContainer } from 'cdbreact-pro';
const Stepper = () => { const [active, setActive] = useState(1);
const handleNextPrevClick = a => { setActive(a); };
const handleSubmission = () => { alert('Form submitted!'); };
return ( <CDBContainer className="d-flex"> <CDBContainer style={{ height: '500px', width: '150px' }}> <CDBStepper direction="vertical"> <CDBStep id={1} far icon="folder-open" name="Basic Information" handleClick={() => handleNextPrevClick(1)} active={active} /> <CDBStep id={2} icon="pencil-alt" name="Personal Data" handleClick={() => handleNextPrevClick(2)} active={active} /> <CDBStep id={3} icon="dragon" name="Terms and Conditions" handleClick={() => handleNextPrevClick(3)} active={active} /> <CDBStep id={4} icon="check" name="Finish" handleClick={() => handleNextPrevClick(4)} active={active} /> </CDBStepper> </CDBContainer> <CDBContainer style={{ height: '500px', width: '100%', display: 'flex', alignItems: 'center', }} > {active === 1 && ( <CDBContainer md="12"> <h3 className="font-weight-bold pl-0 my-4 " style={{ width: '100%', fontSize: '30px', textAlign: 'center', }} > Your Information </h3> <CDBInput label="Email" className="mt-4" /> <CDBInput label="username" className="mt-4" /> <CDBInput label="Password" className="mt-4" /> <CDBInput label="Repeat Password" className="mt-4" /> <CDBBtn color="dark" block flat className="float-right" onClick={() => handleNextPrevClick(2)} > Next </CDBBtn> </CDBContainer> )} {active === 2 && ( <CDBContainer md="12"> <h3 className="font-weight-bold pl-0 my-4" style={{ width: '100%', fontSize: '30px', textAlign: 'center', }} > Personal Data </h3> <CDBInput label="First Name" className="mt-3" /> <CDBInput label="Second Name" className="mt-3" /> <CDBInput label="Surname" className="mt-3" /> <CDBInput label="Address" type="textarea" rows="2" /> <CDBBtn color="light" flat className="float-left" onClick={() => handleNextPrevClick(1)} > Previous </CDBBtn> <CDBBtn color="dark" flat className="float-right" onClick={() => handleNextPrevClick(3)} > Next </CDBBtn> </CDBContainer> )} {active === 3 && ( <CDBContainer md="12"> <h3 className="font-weight-bold pl-0 my-4" style={{ width: '100%', fontSize: '30px', textAlign: 'center', }} > Terms and conditions </h3> <CDBInput label="I agree to the terms and conditions" type="checkbox" id="checkbox3" /> <CDBInput label="I want to receive newsletter" type="checkbox" id="checkbox4" /> <CDBBtn color="light" className="float-left" flat onClick={() => handleNextPrevClick(2)} > Previous </CDBBtn> <CDBBtn color="dark" className="float-right" flat onClick={() => handleNextPrevClick(4)} > Next </CDBBtn> </CDBContainer> )} {active === 4 && ( <CDBContainer md="12"> <h3 className="font-weight-bold pl-0 my-4" style={{ width: '100%', fontSize: '30px', textAlign: 'center', }} > Finish </h3> <h2 className="text-center font-weight-bold my-4">Registration completed!</h2> <CDBBtn color="light" flat className="float-left" onClick={() => handleNextPrevClick(3)} > Previous </CDBBtn> <CDBBtn color="success" flat className="float-right" onClick={handleSubmission}> Submit </CDBBtn> </CDBContainer> )} </CDBContainer> </CDBContainer> );};
export default Stepper;
In the code, we imported some components we need to build a stepper. They include
CDBStepper
: The CDBStepper component holds the steps.CDBStep
: The CDBStep component is nested in the stepper, they indicate the various stages of progression.CDBInput
: The CDBInput component is used to collect info or data from the user.CDBBtn
: The CDBBtn component is used by the user to submit info or interact with the web pages.CDBContainer
: The CDBContainer is used to group, or put other components in a box ( container ).
In the above code, we added styling to the components via the predefined styling we get from the React Bootstrap library, CDBReact using its classNames, and for the native HTML elements like the h3
element, we use inline styling.
Finally, we import the Stepper
Component we just created in our app.js
file to see our stepper in the browser.
import './App.css';import Stepper from './stepper';
function App() { return ( <Router> <div className="App"> <Stepper /> </div> </Router> );}
export default App;
Conclusion
Building a React Bootstrap 5 stepper using CDBReact is quite simple and allows you to use several tools, including bootstrap styling, without installing bootstrap 5.
Resources
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts