How to create a responsive React Bootstrap Sidebar
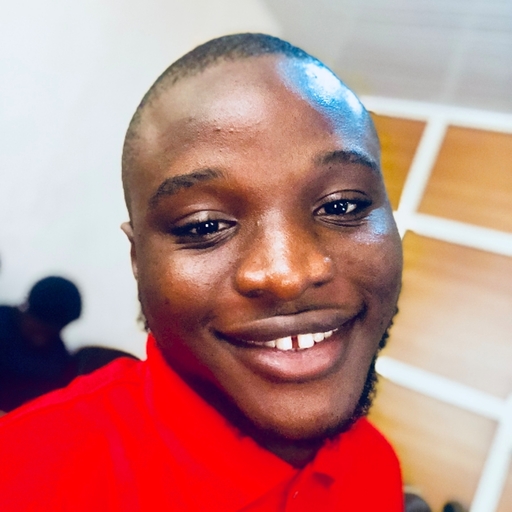
By Chimdia Anyiam
Web Developer
React Boostrap 5 Sidebar
As its name suggests, React Bootstrap 5 Sidebars are usually situated at the side of a webpage, either to the right or the left. You can also add a react-bootstrap sidebar menu collapse to your project. we will be creating a React Bootstrap 5 Sidebar using a react library known as Contrast or CDBReact. This sidebar will help in organizing content and providing easy navigation.
Table of Contents
- Introduction
- What is Sidebar
- How to create Bootstrap 5 sidebars
- Setup and Prerequisities
- Responsive React Bootstrap 5 Sidebar Examples
- Responsive React bootstrap Sidebar
- Responsive React Bootstrap Multilevel Advanced Sidebar
- Conclusion
- Resources
Introduction
In this article, we will create a responsive React Bootstrap Sidebar using the Contrast library (CDBReact). CDBReact is a react library which is an Elegant UI kit with full bootstrap support that has reusable components for building mobile-first, responsive websites and web apps.
What is Sidebar
Sidebars are essential for separating content on a website. They provide space for ads, related posts, navigation links, and more.
Setup and Prerequisites
Prerequisites
Before starting, ensure you have the following:
- Basic knowledge of React
- Basic knowledge of Bootstrap
- Node.js and npm installed
Check Node.js Installation
First, ensure you have Node.js installed. Run the following command in your terminal:
node -v
If Node.js is not installed, download it here. This will also install npm.
Create a React Project
Create a new React project by running:
npx create-react-app sidebar-app
You can name the project whatever you like.
Install CDBReact
Install the Contrast library (CDBReact) in your project:
npm install --save cdbreact
Or using Yarn:
yarn add cdbreact
Install React Router
Our sidebar will use the NavLink component from React Router. Install it by running:
npm install react-router-dom
Wrap App with BrowserRouter
Wrap your app with the BrowserRouter
component from React Router:
import './App.css';import Sidebar from './sidebar';import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"></div> </Router> );}
export default App;
Responsive React Bootstrap 5 Sidebar Examples
Responsive React bootstrap Sidebar
Create a file named sidebar.js
and import the necessary components including some from CDBReact:
import React from 'react';import { CDBSidebar, CDBSidebarContent, CDBSidebarFooter, CDBSidebarHeader, CDBSidebarMenu, CDBSidebarMenuItem,} from 'cdbreact';import { NavLink } from 'react-router-dom';
const Sidebar = () => { return ( <div style={{ display: 'flex', height: '100vh', overflow: 'scroll initial' }}> <CDBSidebar textColor="#fff" backgroundColor="#333"> <CDBSidebarHeader prefix={<i className="fa fa-bars fa-large"></i>}> <a href="/" className="text-decoration-none" style={{ color: 'inherit' }}> Sidebar </a> </CDBSidebarHeader>
<CDBSidebarContent className="sidebar-content"> <CDBSidebarMenu> <NavLink exact to="/" activeClassName="activeClicked"> <CDBSidebarMenuItem icon="columns">Dashboard</CDBSidebarMenuItem> </NavLink> <NavLink exact to="/tables" activeClassName="activeClicked"> <CDBSidebarMenuItem icon="table">Tables</CDBSidebarMenuItem> </NavLink> <NavLink exact to="/profile" activeClassName="activeClicked"> <CDBSidebarMenuItem icon="user">Profile page</CDBSidebarMenuItem> </NavLink> <NavLink exact to="/analytics" activeClassName="activeClicked"> <CDBSidebarMenuItem icon="chart-line">Analytics</CDBSidebarMenuItem> </NavLink>
<NavLink exact to="/hero404" target="_blank" activeClassName="activeClicked"> <CDBSidebarMenuItem icon="exclamation-circle">404 page</CDBSidebarMenuItem> </NavLink> </CDBSidebarMenu> </CDBSidebarContent>
<CDBSidebarFooter style={{ textAlign: 'center' }}> <div style={{ padding: '20px 5px', }} > Sidebar Footer </div> </CDBSidebarFooter> </CDBSidebar> </div> );};
export default Sidebar;
In the file above, we import a few things from CDBReact, such as
- The sidebar itself (CDBSidebar)
CDBSidebarContent
which contains the main part of the sidebarCDBSidebarFooter
which is the footer of the sidebarCDBSidebarHeader
which is the header of the sidebarCDBSidebarMenu
which is the sidebar menuCDBSidebarMenuItem
which is the items that will be added to the sidebar menu.
We also import NavLink
from React-router
Let us import our newly created sidebar component into our app component.
import './App.css';import Sidebar from './sidebar';import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"> <Sidebar /> </div> </Router> );}
export default App;
At this point, your sidebar should look like the images below.
With this, we have successfully created the React boostrap 5 sidebar and can now use it as navigation to different parts of our website or add other content to it as required.
Responsive React Bootstrap Multilevel Advanced Sidebar
To create a responsive bootstrap multilevel advanced sidebar, you will need the pro version of Contrast. Get Contrast Pro here.
We use the pro-react-bootstrap 5 sidebars the same way we use the free React bootstrap 5 sidebars. After downloading the files for the contrast pro package, you can get by clicking the link above, you follow these steps to get the multilevel advanced sidebar working.
Install CDBReact-pro
Install the cdbreact-pro package in your project (we recommend adding the file to the root of the project.) by running.
npm install --save ./path-to-the-cdbreact-pro-tgz-file
Or using Yarn
yarn add ./path-to-the-cdbreact-pro-tgz-file
Our Multilevel Advanced Sidebar would also be using the Navlink component from React router that we installed above.
Now restart the server by running npm start
to ensure that everything works well and there are no errors.
Creating the multilevel Sidebar
Before we proceed, let’s go ahead and wrap our app with the BrowserRouter component from react-router-dom as Navlinks can’t work outside it.
import './App.css';import Sidebar from './sidebar';import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"></div> </Router> );}export default App;
Let us go ahead to create a file named prosidebar.js
which would contain our Prosidebar component. Import the various sidebar components that we’ll be using.
import React from 'react';import { CDBBadge, CDBSidebar, CDBSidebarContent, CDBSidebarFooter, CDBSidebarHeader, CDBSidebarMenu, CDBSidebarMenuItem, CDBSidebarSubMenu,} from 'cdbreact-pro';import { NavLink } from 'react-router-dom';
const Sidebar = () => { return ( <div style={{ display: 'flex', height: '100vh', overflow: 'scroll initial' }}> <CDBSidebar textColor="#fff" backgroundColor="#333"> <CDBSidebarHeader prefix={<i className="fa fa-bars fa-large"></i>}> <a href="/" className="text-decoration-none" style={{ color: 'inherit' }}> Sidebar </a> </CDBSidebarHeader>
<CDBSidebarContent> <CDBSidebarMenu> <CDBSidebarMenuItem suffix={ <CDBBadge color="danger" size="small" borderType="pill"> new </CDBBadge> } icon="th-large" > Dashboard </CDBSidebarMenuItem> <CDBSidebarMenuItem icon="sticky-note" suffix={ <CDBBadge color="danger" size="small" borderType="pill"> new </CDBBadge> } > Components </CDBSidebarMenuItem> </CDBSidebarMenu> <CDBSidebarMenu> <CDBSidebarSubMenu title="Sidemenu" icon="th"> <NavLink exact to="/sub1" activeClassName="activeClicked"> <CDBSidebarMenuItem>submenu 1</CDBSidebarMenuItem> </NavLink> <NavLink exact to="/sub2" activeClassName="activeClicked"> <CDBSidebarMenuItem>submenu 2</CDBSidebarMenuItem> </NavLink> <NavLink exact to="/sub3" activeClassName="activeClicked"> <CDBSidebarMenuItem>submenu 3</CDBSidebarMenuItem> </NavLink> </CDBSidebarSubMenu> <CDBSidebarSubMenu title="Sidemenu2" icon="book" suffix={ <CDBBadge color="danger" size="small" borderType="pill"> new </CDBBadge> } > <CDBSidebarMenuItem>submenu 1</CDBSidebarMenuItem> <CDBSidebarMenuItem>submenu 2</CDBSidebarMenuItem> <CDBSidebarMenuItem>submenu 3</CDBSidebarMenuItem> </CDBSidebarSubMenu> <CDBSidebarSubMenu title="MultiLevel with Icon" icon="table"> <CDBSidebarMenuItem>submenu 1 </CDBSidebarMenuItem> <CDBSidebarMenuItem>submenu 2 </CDBSidebarMenuItem> <CDBSidebarSubMenu title="submenu 3"> <CDBSidebarMenuItem>submenu 3.1 </CDBSidebarMenuItem> <CDBSidebarMenuItem>submenu 3.2 </CDBSidebarMenuItem> <CDBSidebarSubMenu title="subnet"> <CDBSidebarMenuItem>submenu 3.3.1 </CDBSidebarMenuItem> <CDBSidebarMenuItem>submenu 3.3.2 </CDBSidebarMenuItem> <CDBSidebarMenuItem>submenu 3.3.3 </CDBSidebarMenuItem> </CDBSidebarSubMenu> </CDBSidebarSubMenu> </CDBSidebarSubMenu> </CDBSidebarMenu> </CDBSidebarContent>
<CDBSidebarFooter style={{ textAlign: 'center' }}> <div style={{ padding: '20px 5px', }} > Sidebar Footer </div> </CDBSidebarFooter> </CDBSidebar> </div> );};
export default Sidebar;
In the file above, we import a few things from CDBReactPro, such as
- The sidebar itself (CDBSidebar)
CDBSidebarContent
which contains the main part of the sidebarCDBSidebarFooter
which is the footer of the sidebarCDBSidebarHeader
which is the header of the sidebarCDBSidebarMenu
which is the sidebar menuCDBSidebarMenuItem
which is the items that will be found on the sidebar menu andCDBSidebarSubmenu
which is the sidebar sub-menu.
We also imported NavLink from React-router. Then we went ahead to include the sidebar header, footer and some inline styles to these components. The body content was aslso added to complete the react bootstrap multilevel sidebar.
Import ProSidebar into App Component
Import the ProSidebar component into your app component:
import './App.css';import Sidebar from './sidebar';import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"> <Sidebar /> </div> </Router> );}
export default App;
Is There a Sidebar in Bootstrap 5?
Out of the box, Bootstrap 5 does not contain a sidebar component. Bootstrap's grid system, utilities (especially those for flex), and components like nav or collapse are not sidebar-specific, but you can make something that looks acceptable there.
Bootstrap Vertical Side Menu
In Bootstrap, a vertical side menu is an example of one ascribed type navigation component that placed vertically on the left or right of webpage. This typically includes links to different parts of the site and may be styled with Bootstrap classes.
Conclusion
By following this guide, you should now have a fully functional, responsive React Bootstrap sidebar. Whether it's a simple sidebar or a multilevel one, having this feature will definitely add value to your website or web application.
Resources
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts