How to create a beautiful React Bootstrap select with icons.
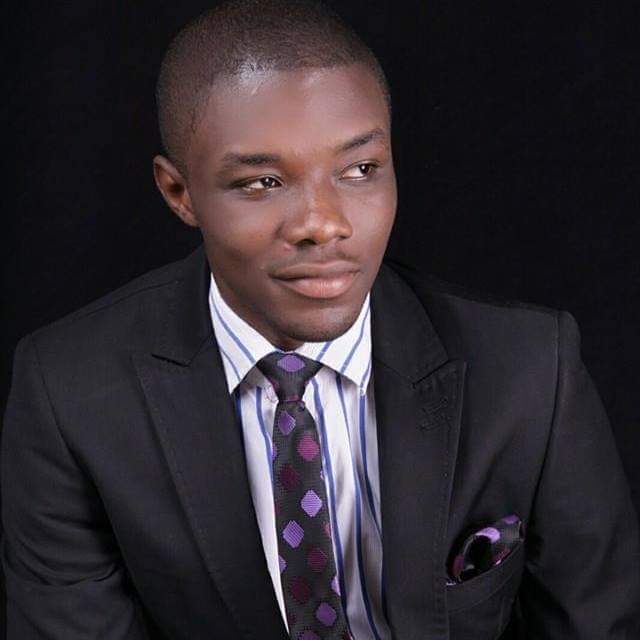
By Emmanuel Chinonso
Web Developer
Ract Bootstrap 5 Select
The React Bootstrap Select component is a form control that displays a collapsible list of multiple values, which can be used in forms, menus, or surveys after the click. Select enables you to use ↑
↓
arrow keys to navigate through options and use the ↵
key to select the required option (works for stateful select). We are going to look at how to create a selection using Contrast(CDBReact).
Table of Contents
- Prerequisites
- Setup
- Installing CDBReact Pro
- Creating the Select Component
- Integrating the Select Component
- How to Use React Bootstrap Select
- Resources
Prerequisites
The react Bootstrap Select would be built using React, Bootstrap, and CDBReact. You don’t need to have any previous knowledge of CDBReact, but the following are necessary:
- JavaScript Knowledge
- Basic React Knowledge
- Basic Bootstrap knowledge
- NPM installed
The select page with the icon we are going to build looks like the image below
Setup
First, Check that you have the node installed. To do this, run the following command in your terminal:
node-v
This should show you the current version of the node you have installed on your machine. If you don’t have a node installed, download it here. Installing node also installs npm on your PC.
Now that we have the node installed, we can start up our React project by going to the directory of our choice and running.
npx create-react-app select-app
I named the project select-app
, but you can use whatever name of your choice.
Installing CDBReact-pro
Before we install our CDBReact we have to download the pro version here first. Then, we can go ahead to install them in our project. It is advised to add the file to the root of the project by running:
npm install --save ./path-to-the-cdbreact-pro-tgz-file
Or using Yarn
yarn add ./path-to-the-cdbreact-pro-tgz-file
Note we don’t need to install bootstrap or add it anywhere in our project as CDBReact does that for us upon installation.
import React, { useState } from 'react';import { CDBSelect, CDBContainer } from 'cdbreact-pro';
export const Select = () => { const [option] = useState([ { text: 'Select Option', icon: 'stack-overflow', }, { text: 'Another Option', icon: 'reddit', }, { text: 'Option 3', icon: 'instagram', }, { text: 'Option 4', }, { text: 'Last Option', }, ]); return ( <CDBContainer> <CDBSelect2 options={option} iconBrand selected="Choose an option" color="white" /> </CDBContainer> );};
export default Select;
The select component is created in the above code, and the various styles and icons are added. Let us import the created select component into our app component.
import './App.css';import Select from './select';import Reactdom from 'react-dom';
function App() { return ( <div className="App"> <Select /> </div> );}
Reactdom.render(<App />, document.getElementById('root'));
Our page should look like this
Conclusion
A React Bootstrap 5 select using CDBReact is quite simple to build and allows you to use several tools, including bootstrap styling without installing bootstrap to create your select with icons.
Resources
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts