React Bootstrap Progress bar-How to create a beautiful React Bootstrap 5 progress bar.
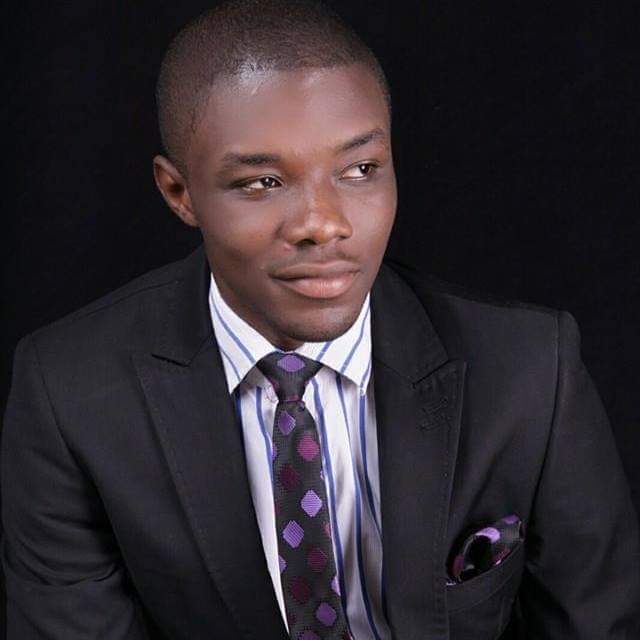
By Emmanuel Chinonso
Web Developer
React Bootstrap 5 Progress Bar
Progress bars are essential UI components that visually indicate the progress of a process or activity. In this tutorial, we'll explore how to create both linear and circular progress bars using Contrast or CDBReact which is built with React Boostrap 5.
Contrast, also known as CDBReact or React Bootstrap, is a react library which is an Elegant UI kit with full bootstrap support that has reusable components for building mobile-first, responsive websites and web apps.
Prerequisites Before diving into the progress bar implementation, ensure you have:
- Basic knowledge of JavaScript and React
- Node.js and npm installed on your computer
You don’t need to have any previous knowledge of CDBReact.
Setting Up Your React Environment
Start by setting up a new React project if you haven't already:
npx create-react-app progressbar-appcd progressbar-app
Installing React Bootstrap and CDBReact
React Bootstrap simplifies the integration of Bootstrap components into your React projects. Install it along with CDBReact for additional custom components:
npm install react-bootstrap cdbreact
Or using Yarn
yarn install react-bootstrap cdbreact
Note that we don’t need to install bootstrap or add it anywhere in our project as CDBReact does that for us upon installation.
React Bootstrap Progress Bar Examples
This section will showcase various examples of progress bars that can be implemented using React Bootstrap 5 and CDBReact.
Circular progress bar
Let us go ahead to create a file named circularpro.js
which would contain our progress bar component. Import the various progress bar components that we will need from CDBReact.
import React from 'react';import { CDBCircularProgress, CDBContainer } from 'cdbreact-pro';
In the code above, we import React from react and two components from the CDBReact, which are:
- CDBContainer
- CDBCircularProgress.
export const Progress = () => { return ( <CDBContainer> <CDBCircularProgress value={25} max={100} min={0} text={`${25}%`} /> <CDBCircularProgress value={50} max={100} min={0} text={`${50}%`} color="primary" /> <CDBCircularProgress value={95} max={100} min={0} color="secondary" text={`${95}%`} /> <CDBCircularProgress value={35} max={100} min={0} color="danger" text={`${35}%`} /> <CDBCircularProgress value={47} max={100} min={0} color="info" text={`${47}%`} /> <CDBCircularProgress value={100} max={100} min={0} color="success" text={`${100}%`} /> </CDBContainer> );};
export default Progress;
We added some styling to the react-bootstrap circular progress bar in the code above to make it look appealing.
Now, we can render the component in app.js
.
import './App.css';import tab from './ circularpro.js ';import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"> <Progress /> </div> </Router> );}
export default App;
Preview
Linear progress bar
For the react bootstrap 5 linear progress bar, we will create a file named linearpro.js
which would contain our progress bar component.
Go ahead to Import the various progress bar components that we will need from CDBReact.
import React from 'react';import { CDBProgress, CDBContainer } from 'cdbreact-pro';
the code above imported React from react, the CDBProgress, and the CDBContainer from cdbreact.
export const Progress = () => { return ( <CDBContainer> <CDBProgress value={10} text={`${10}%`} colors="primary" /> <CDBProgress value={20} text={`${20}%` colors="secondary" /> <CDBProgress value={70} text={`${70}%`} colors="success" /> <CDBProgress value={40} text={`${40}%`} colors="danger" /> <CDBProgress value={90} text={`${90}%`} colors="info" /> <CDBProgress value={60} text={`${60}%`} colors="warning" /> <CDBContainer/> );}export default Progress;
This code adds features to the linear progress bar and is enclosed in a CDBContainer component from contrast.
import './App.css';import tab from './ linearpro.js ';import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"> <Progress /> </div> </Router> );}export default App;
in this code, we rendered our linear progress bar in our app.js
file.
Preview
Conclusion
Integrating React Bootstrap 5 progress bars into your applications not only enhances the user interface but also improves the overall user experience by providing clear visual cues about process completions. Follow this guide to implement stylish and responsive progress bars in your next project using CDBReact.
Resources
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts