How to use Bootstrap in React Using a React Library
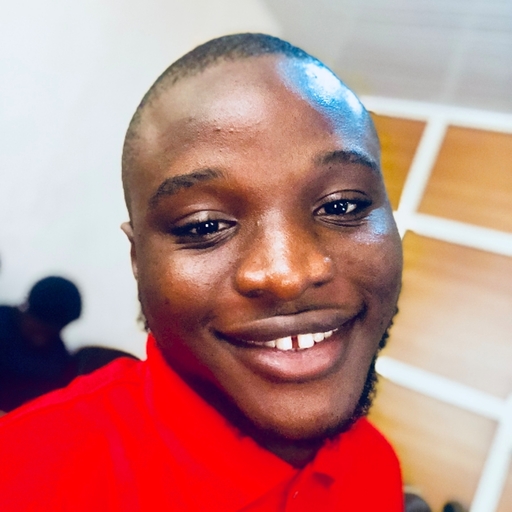
By Chimdia Anyiam
Web Developer
Bootstrap in React
React is one of the most popular javascript frontend frameworks out there and its popularity does not come as a surprise as it is very flexible to use. Bootstrap on the other hand is an open-source CSS framework that features many templates for user interface components such as cards and modals.
In this article, we will be making use of these two frameworks alongside a react library to create a simple landing page. The library we’ll be making use of is CDBReact which is an Elegant UI kit that has reusable components for building mobile-first, responsive websites, and web apps.
Prerequisites
The Landing page would be built with React, Bootstrap, and CDBReact. You don’t need to have any previous knowledge of CDBReact.
- Basic React Knowledge
- Basic Bootstrap knowledge
Node
and its package manager,npm
. You can install it from. Run the command
node -v
&&npm -v
to verify you have them installed.- Alternatively, we can use another package manager,
.
This is an image of the Landing page that we will build.
Setup
First Check that you have the node installed. To do this, run the following code
node - v;
If you don’t have node.js installed, download it here
Installing node also installs npm on your PC but you can still confirm using
npm -v.
Now that we have node installed, we can start up our React project by going to the directory of our choice and running
npx create-react-app landing-page
I chose a landing page for the name of my project but you can use whatever you want.
Install cdbreact
Now, we have to install cdbreact in our project
npm install --save cdbreact
Or using Yarn
yarn add cdbreact
Note that we don't need to install bootstrap or add it anywhere in our project as CDBReact does that for us upon installation.
Also, Install React router because it is required by the Navbar we’ll be creating.
npm install react-router-dom
Now run npm start
to make sure that everything runs smoothly
Navbar
Now let’s proceed to create a navbar for our landing page. Create a file named Navbar. Import Navbar and other components we will be using from cdbreact
import React, { useState } from 'react';
import { CDBBtn, CDBNavbar, CDBNavBrand, CDBNavbarNav, CDBNavToggle, CDBNavItem, CDBNavLink, CDBCollapse,} from 'cdbreact';
After that, add the following code for the navbar.
const Navbar = () => { const [collapse, setCollapse] = useState(false);
return ( <header className="page-header" style={{ width: '60%', margin: '0 auto', 'max-width': '1320px' }} > <CDBNavbar className="navigation bg-transparent p-0" expand="md" dark scrolling> <CDBNavBrand href="/"> <img alt="logo" src="/logo192.png" width="25px" /> </CDBNavBrand>
<CDBNavToggle onClick={() => { setCollapse(!collapse); }} />
<CDBCollapse id="navbarCollapse1" delay="0" isOpen={collapse} navbar> <CDBNavbarNav> <CDBNavItem active> <CDBBtn flat className="p-2 border-0 bg-transparent"> <CDBNavLink to="#">Home</CDBNavLink> </CDBBtn> </CDBNavItem>
<CDBNavItem> <CDBBtn flat className="p-2 border-0 bg-transparent"> <CDBNavLink to="#">Resources</CDBNavLink> </CDBBtn> </CDBNavItem>
<CDBNavItem> <CDBBtn flat className="p-2 border-0 bg-transparent"> <CDBNavLink to="#">Blog</CDBNavLink> </CDBBtn> </CDBNavItem>
<CDBNavItem> <CDBBtn flat className="p-2 border-0 bg-transparent"> <CDBNavLink to="#">Contact</CDBNavLink> </CDBBtn> </CDBNavItem>
<CDBNavItem> <CDBBtn flat className="p-2 border-0 bg-transparent"> <CDBNavLink to="#">Support</CDBNavLink> </CDBBtn> </CDBNavItem> </CDBNavbarNav> </CDBCollapse> </CDBNavbar> </header> );};
export default Navbar;
From the piece of code above, we imported the necessary components from cdbreact and then we also created a collapsed state that will be used by the navbar to know when it is open or not. We can notice some bootstrap classes such as p-2
and border-0
being used in the styling of the navbar.
Let’s import the Navbar into our App.js and also include our router from react-router-dom.
import './App.css';
import Navbar from './Navbar';
import { BrowserRouter as Router } from 'react-router-dom';
function App() { return ( <Router> <div className="App"> <Navbar /> </div> </Router> );}
export default App;
Now let's edit our App.css file to give our page a black background color
.App { background-color: black;}
After this, your navbar should appear like in the image below.
Now let's move on to creating the rest of the page.
Landing Component
Create a new file called landing.js and in it, create a component called landing, also create a landing.css file that would be used for styling.
In your Landing.js, add the following code
import React from 'react';
import './landing.css';
import { CDBBtn } from 'cdbreact';
const Landing = () => { return ( <section className="page-head d-flex align-items-center text-right text-white"> <img alt="landing" src="/landing.png" className="image" />
<div className="page-info"> <p className="page-title font-weight-bold ml-auto">Creativity is but skin deep</p>
<p className="my-4"> Lorem ipsum dolor sit amet, consectetur adipisicing elit. Fugit, error amet numquam iure provident voluptate esse quasi, veritatis totam voluptas nostrum quisquam eum porro a pariatur accusamus veniam. </p>
<CDBBtn flat style={{ background: '#8080ff', border: '2px #8080ff solid' }}> Start Now </CDBBtn> </div> </section> );};
export default Landing;
Here we import the landing.css file, we also import CDBBtn component from CDBReact, and then use several bootstrap classes such as d-flex
, align-items-center
, and my-4
for styling. Also notice that we can pass custom styles to CDBReact components as we did to CDBBtn
above.
Also, add the following to your landing.css file.
.page-head { margin: 5rem auto 0 auto; width: 80%;}
.page-head .image { width: 50%; max-width: 550px;}
.page-info { width: 50%; text-align: right;}
.page-title { font-size: 3em; max-width: 350px;}
.page-info .btn { max-width: 200px;}
Then import the landing component into your App.js file, to make it look like this:
import './App.css';
import Navbar from './Navbar';
import { BrowserRouter as Router } from 'react-router-dom';
import Landing from './landing';
function App() { return ( <Router> <div className="App"> <Navbar /> <Landing /> </div> </Router> );}
export default App;
Now your landing page should be ready and look like the image below.
Congratulations, you have successfully built a landing page using react, bootstrap and CDBReact. Easy isn’t it? Pairing these tools can help you create awesome web pages that look beautiful in no time.
Resources
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts