Tutorial: Javascript Variables
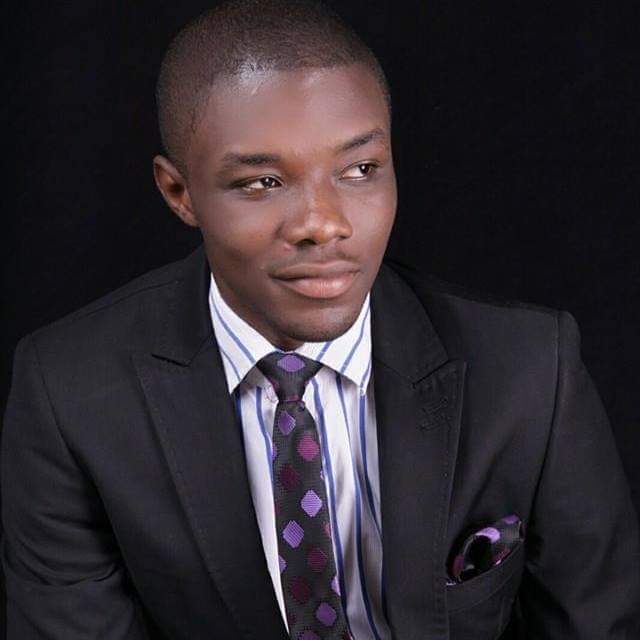
By Emmanuel Chinonso
Web Developer
JavaScript Variables
In JavaScript, variables are data storage containers. A variable must be declared before it can be used in a JavaScript program. The var keyword is used to declare variables, as shown below.
Code:
- var x = 5;
- var y = 6;
- var z = x + y;
Variables x, y, and z are declared with the var keyword in this example. As a result of the above example, you can anticipate:
- x holds the value 5
- y holds the value 6 and
- z holds the value 11.
Declaring all variables at the start of a script is good programming practice.
JavaScript Identifiers
All variables in JavaScript must have distinct names. Identifiers are the names given to these distinct names. Short names (like x and y) or more descriptive names can be used as identifiers (age, sum, totalVolume). The following are the general guidelines for naming variables (unique identifiers):
- Letters, numerals, underscores, and dollar signs can all be used in names.
- Each name must start with a letter.
- Names can also start with the letters \$ or .
- Case is important while typing names (y and Y are different variables)
- Names containing reserved terms (such as JavaScript keywords) are not allowed.
There are a lot of variables in a single statement.
Many variables can be declared in a single sentence. Start the statement with var and use a comma to separate the variables:
Code:
var person = 'John Doe', carName = 'Volvo', price = 200;
A declaration can span multiple lines:
Code :
var person = 'John Doe', carName = 'Volvo', price = 200, Value = undefined;
Variables are frequently declared in computer programs without a value. The value can be calculated or provided later, such as user input. The value of a variable declared without a value is undefined. Following the execution of this statement, the variable carName will have the value undefined:
Code :
var carName;
Re-Declaring JavaScript Variables:
A JavaScript variable's value will not be lost if you re-declare it. Following the execution of these statements, the variable carName will still have the value "Volvo".
Code:
var carName = 'Volvo';var carName;
Strings can also be added, but they will be concatenated:
Code:
var x = 'John' + ' ' + 'Doe';
If you put a number in quotes, it will be interpreted as a string and concatenated with the rest of the numbers.
JavaScript Dollar Sign $
Remember that identifiers (names) in JavaScript must begin with:
- An alphabetical letter (A-Z or a-z)
- A dollar symbol "\$"
- A dollar symbol "\$"
Because the dollar sign is treated as a letter in JavaScript, identifiers containing \$ are valid variable names.
Code:
var $$$ = 'Hello World';var $ = 2;var $myMoney = 5;
Although the dollar symbol is not widely used in JavaScript, professional programmers frequently use it as an alias for a JavaScript library's main function.
The main function $ in the JavaScript library jQuery, for example, is used to select HTML elements. $("p"); in jQuery indicates "select all p elements".
JavaScript Underscore _
Because the underscore is treated as a letter in JavaScript, identifiers containing are valid variable names.
Code:
var _lastName = 'Johnson';var _x = 2;var _100 = 5;
Although using the underscore as another name for private (hidden) variables is uncommon in JavaScript, it is a regular practice among skilled programmers.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts