Tutorial: Javascript This Keyword
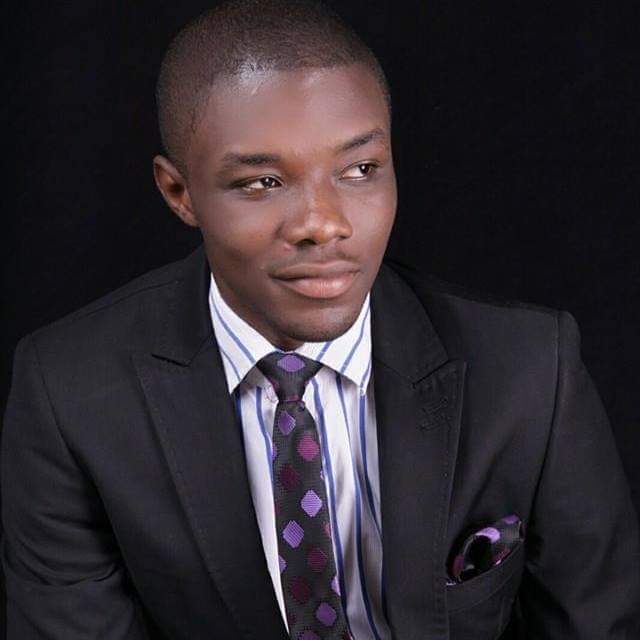
By Emmanuel Chinonso
Web Developer
The JavaScript this Keyword
var person = { firstName: 'John', lastName: 'Doe', id: 5566, fullName: function() { return this.firstName + ' ' + this.lastName; },};
What is this?
This Keyword in JavaScript refers to object that it belongs to. it has different values based on where it is used:
When This is used in method, it refers to the owner object.
This refers to the global object when used alone.
-This refers to the global object in a function.
when used in strict mode, this keyword is undefined in a function.
This is the element that got the event in an event.
Any object can be referenced using methods like call() and apply().
this in a Method
This refers to the method's "owner" in an object method. This refers to the person object in the code at the top of the page. The fullName method belongs to the person object.
fullName : function() { return this.firstName + " " + this.lastName;}this Alone
The Global object is the owner when used alone, hence this refers to the Global object. The Global object in a browser window is [object Window]:
JavaScript Code:
var x = this;
This also relates to the Global object [object Window] in strict mode when used alone:
JavaScript Code:
'use strict';var x = this;
this in a Function (Default)
In a JavaScript function, the function's owner is the default binding for this keyword. The Global object [object Window] in a function is referred to when using this keyword.
JavaScript Code:
function myFunction() { return this;}
this in a Function (Strict)
The strict mode of JavaScript prevents default binding. As a result, when used in strict mode in a function, this is undefined. "Make use of strict";
function myFunction() { return this;}
this in Event Handlers
This is the HTML element that received the event in HTML event handlers:
JavaScript Code:
<button onclick="this.style.display='none'">Click to Remove Me!</button>
Object Method Binding
This is the person object in this code (the person object is the "owner" of the function): JavaScript Code:
var person = { firstName: 'John', lastName: 'Doe', id: 5566, myFunction: function() { return this; },};
JavaScript Code:
var person = { firstName: 'John', lastName: 'Doe', id: 5566, fullName: function() { return this.firstName + ' ' + this.lastName; },};
To put it another way: this.firstName means the firstName property of this (person) object.
Explicit Function Binding
The call() and apply() methods are JavaScript methods that are predefined. They can both be used to invoke an object method with an argument of another object. Later in this course, you'll learn more about call() and apply(). When invoking person1.fullName with the argument person2, even though it is a method of person1, it will refer to person2:
JavaScript Code:
var person1 = { fullName: function() { return this.firstName + ' ' + this.lastName; },};var person2 = { firstName: 'John', lastName: 'Doe',};person1.fullName.call(person2); // Will return "John Doe"
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts