Tutorial: Javascript String Methods
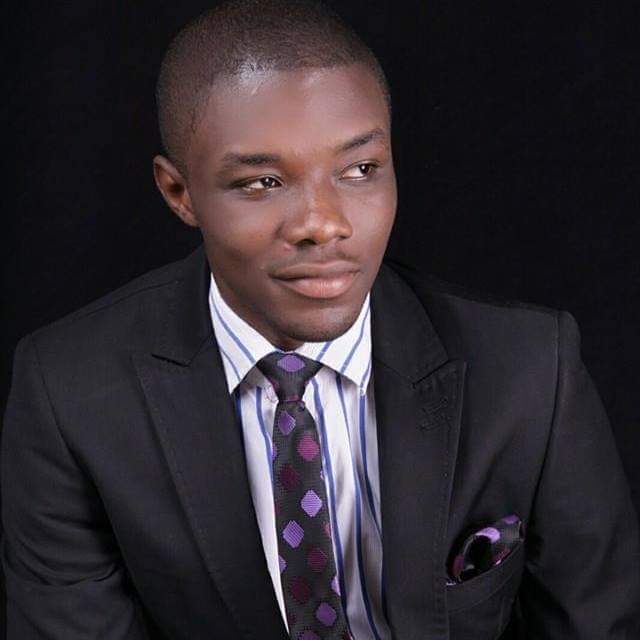
By Emmanuel Chinonso
Web Developer
JavaScript String Methods
You can use string methods to work with strings.
String Methods and Properties Values with no properties or methods, such as "John Doe", are considered primitive (because they are not objects). However, primitive values can use JavaScript methods and properties because JavaScript interprets primitive values as objects when executing methods and properties.
String Length: The length attribute returns the string's length.
JavaScript Code:
var txt = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ';var alpha = txt.length;
Finding a String in a String:
The indexOf() method returns the position of the first occurrence of a string's provided text. JavaScript starts counting at zero. In a string, 0 is the first place, 1 is the second, 2 is the third ...
JavaScript Code:
var str = "Please locate where 'locate' occurs!";var pos = str.indexOf('locate');
The lastIndexOf() method returns the index of a string's last occurrence of a given text:
JavaScript Code:
var str = "Please locate where 'locate' occurs!";var pos = str.lastIndexOf('locate');
If the text cannot be retrieved, both indexOf() and lastIndexOf() return -1. Both methods take a second parameter as the search's starting point:
JavaScript Code:
var str = "Please locate where 'locate' occurs!";var pos = str.indexOf('locate', 15);
The lastIndexOf() method searches backwards (from the end to the beginning), which means that if the second parameter is 15, the search will begin at position 15 and continue until the string reaches the beginning.
JavaScript Code:
var str = "Please locate where 'locate' occurs!";var pos = str.lastIndexOf('locate', 15);
Searching for a String in a String
The search() method looks for a provided value in a string and returns the position of the match.
JavaScript Code:
var str = "Please locate where 'locate' occurs!";var pos = str.search('locate');
Is there a difference between indexOf() and search()? They both take the same arguments (parameters) and produce the same result, right? The two approaches are not equivalent. These are the distinctions:
A second start position argument cannot be passed to the search() method. The indexOf() technique is unable to handle large search values (regular expressions).
Extracting String Parts
There are three methods to remove a part of a string:
- slice(start, end)
- substring(start, end)
- substr(start, length)
The slice() Method
slice() takes a portion of a string and returns it as a new string. The technique accepts two parameters: the start and end positions (end not included). This example cuts a section of a string from position 7 to position 12 (13-1) as follows:
JavaScript Code:
var str = 'Apple, Banana, Kiwi';var res = str.slice(7, 13);
The position is counted from the end of the string if a parameter is negative.
The substring() Method
substring() is similar to slice().The difference is that substring() cannot accept negative indexes.
JavaScript Code:
var str = 'Apple, Banana, Kiwi';var res = str.substring(7, 13);
If you omit the second parameter, substring() will slice out the rest of the string.
The substr() Method
slice()Â is comparable to substr(). The second option, on the other hand, sets the length of the extracted section.
JavaScript Code:
var str = 'Apple, Banana, Kiwi';var res = str.substr(7, 6);
The result of res will be: Banana Substr() will slice out the rest of the string if the second parameter is omitted.
JavaScript Code:
var str = 'Apple, Banana, Kiwi';var res = str.substr(7);
The result of res will be Banana, Kiwi If the first parameter is negative, the position counts from the end of the string.
JavaScript Code:
var str = 'Apple, Banana, Kiwi';var res = str.substr(-4);
The result of res will be: Kiwi
The concat() Method: concat() joins two or more strings:
JavaScript Code:
var text1 = 'Hello';var text2 = 'World';var text3 = text1.concat(' ', text2);
Instead of using the plus operator, you can use the concat() function. These two lines perform the same function.
JavaScript Code:
var text = 'Hello' + ' ' + 'World!';var text = 'Hello'.concat(' ', 'World!');
A new string is returned by all string functions. They don't change the original string in any way. Strings are immutable, according to the formal definition: Strings can only be substituted, not modified.
String.trim() The trim() method removes whitespace from both sides of a string:
JavaScript Code:
var str = ' Hello World! ';alert(str.trim());
If you need to support IE 8, you can use replace() with a regular expression instead
JavaScript Code:
var str = ' Hello World! ';alert(str.replace(/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g, ''));
You can also use the replace solution above to add a trim function to the JavaScript String.prototype:
JavaScript Code:
if (!String.prototype.trim) { String.prototype.trim = function() { return this.replace(/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g, ''); };}var str = ' Hello World! ';alert(str.trim());
Extracting String Characters
There are 3 methods for extracting string characters:
- charAt(position)
- charCodeAt(position)
- The charAt() Method
The charAt() method returns the character at a specified index (position) in a string:
JavaScript Code:
var str = 'HELLO WORLD';str.charAt(0); // returns H
The charCodeAt() Method
The unicode of the character at a particular index in a string is returned by the charCodeAt() method: A UTF-16 code is returned by this procedure (an integer between 0 and 65535).
JavaScript Code:
var str = 'HELLO WORLD';str.charCodeAt(0); // returns 72
Converting a String to an Array
The split() method can be used to convert a string to an array: If the separator isn't specified, the returned array will have the entire string at index [0]. The returned array will be an array of single characters if the separator is "":
JavaScript Code:
var txt = 'a,b,c,d,e'; // Stringtxt.split(','); // Split on commastxt.split(' '); // Split on spacestxt.split('|'); // Split on pipe.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts