Tutorial: Javascript statement, syntax and comments
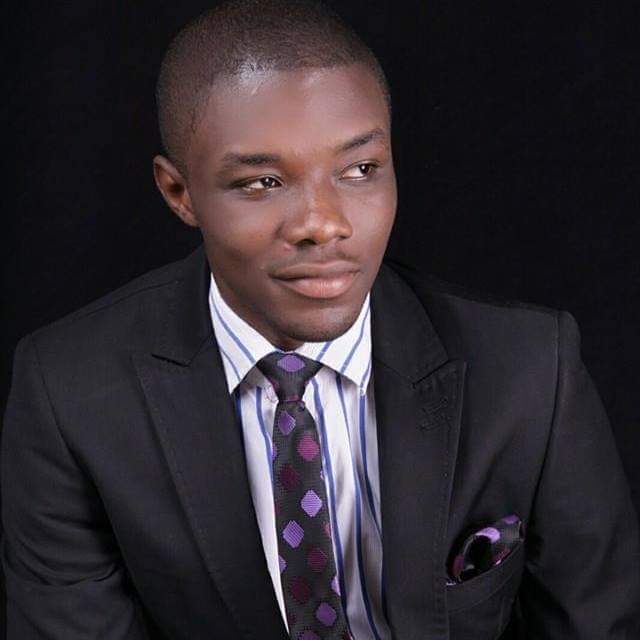
By Emmanuel Chinonso
Web Developer
JavaScript Statement, Syntax and Comments
A computer program is a set of "instructions" that a computer will "execute". These computer instructions are known as statements in a programming language. A list of programming statements makes up a JavaScript program.
Values, Operators, Expressions, Keywords, and Comments make up JavaScript statements. Many JavaScript statements may be found in most JavaScript apps. The assertions are performed in the same order as they are written, one by one. JavaScript statements are separated by semicolons.
JavaScript code:
var a, b, c; // Declare 3 variablesa = 5; // Assign the value 5 to ab = 6; // Assign the value 6 to bc = a + b; // Assign the sum of a and b to c
Multiple statements on a single line are permitted when separated by semicolons. It is not necessary, but highly suggested, to terminate statements with a semicolon. Multiple spaces are ignored by JavaScript. You may make your script more legible by adding white space.
Javascript Code:
var person = 'Hege';var person = 'Hege';
Leaving space around operators is a smart idea. ( = + - * / ):
JavaScript Line Length and Line Breaks
Programmers want to keep code lines under 80 characters long for readability. If a JavaScript statement is too long to fit on a single line, break it after an operator.
Code:
document.getElementById('demo').innerHTML = 'Hello Dolly!';
JavaScript Code Blocks Inside curly braces{...}, JavaScript statements can be grouped together in code blocks. The goal of code blocks is to establish a set of statements that will be executed at the same time. Statements clustered together in blocks can be found in JavaScript functions, for example:
JavaScript Code:
function myFunction() { document.getElementById('demo1').innerHTML = 'Hello Dolly!'; document.getElementById('demo2').innerHTML = 'How are you?';}
JavaScript syntax
The JavaScript syntax is a set of principles for writing JavaScript programs. The JavaScript syntax distinguishes between two kinds of values:
- Fixed values
- Variable values Fixed values are called Literals. Variable values are called Variables.
JavaScript Literals
The following are the two most significant syntactic rules for fixed values:
- Decimals are used with or without numbers.
- Text enclosed in double or single quotations is referred to as a string: Eg "Johnny Smith" 'Johnny Smith'
Actions to be done are identified using JavaScript keywords. The var keyword instructs the browser to create variables in the following way:
Code:
var x, y;x = 5 + 6;y = x * 10;
The var keyword instructs the browser to create variables in the document:
JavaScript Identifiers:
Names are identifiers. Variable names in JavaScript are given using identifiers (and keywords, and functions, and labels). In most computer languages, the regulations for legal names are similar.
- The initial character in JavaScript must be a letter, underscore (_), or dollar sign (\$).
- Letters, numerals, underscores, or dollar signs can be used as subsequent characters.
- As the first character, numbers are not permitted. This allows JavaScript to distinguish between IDs and numbers. The case of all JavaScript identifiers is important. The variables lastName and lastname are not the same thing:
Code
var lastname, lastName;lastName = 'Doe';lastname = 'Peterson';
JavaScript Syntax The JavaScript syntax is a set of principles that describe how JavaScript applications are built:
var x, y, z; // Declare Variablesx = 5;y = 6; // Assign Valuesz = x + y; // Compute Values
JavaScript Values The JavaScript syntax distinguishes between two kinds of values:
- Fixed values also called Literals.
- Variable values also called Variables.
JavaScript Literals: The following are the two most significant syntactic rules for fixed values:
- Decimals or no decimals are used to write numbers:
--- 10.50, 1001
- Text enclosed in double or single quotations is referred to as a string: --- "John Doe", 'John Doe'
JavaScript Variables: Variables are used to hold data values in computer languages.
The var keyword is used in JavaScript to declare variables.
When assigning values to variables, the equal sign is utilized.
The variable x is defined as a variable in this example. The value 6 is then allocated (given) to x:
var x;x = 6;
JavaScript Operators To compute values, JavaScript employs arithmetic operators (+ - * /):
(5 + 6) * 10
To assign values to variables in JavaScript, use the assignment operator (=):
var x, y;x = 5;y = 6;
JavaScript Expressions
An expression is a set of values, variables, and operators that produces a result.
An evaluation is the name given to the process of computing.
For example, 5 * 10 evaluates to 50:
5 * 10
Expressions can also contain variable values:
x * 10
Values can be numbers or strings of various types.
For example, "John" + " " + "Doe", evaluates to "John Doe":
"John" + " " + "Doe"
JavaScript Keywords Actions to be done are identified using JavaScript keywords.
The var keyword instructs the browser to create variables in the document:
var x, y;x = 5 + 6;y = x * 10;
JavaScript Comments
avaScript statements aren't all "executed."
Comments are regarded as code following double slashes / or between / and /.
Comments will be ignored and will not be carried out:
var x = 5; // I will be executed
// var x = 6; I will NOT be executed
Below you will find more information on comments.
JavaScript Identifiers
Names are identifiers.
Variable names in JavaScript are given using identifiers (and keywords, and functions, and labels).
In most computer languages, the regulations for legal names are similar.
The initial character in JavaScript must be a letter, underscore (_), or dollar sign (\$).
Letters, numerals, underscores, and dollar signs can all be used as subsequent characters.
As the first character, numbers are not permitted. This allows JavaScript to distinguish between IDs and numbers.
JavaScript has a case-sensitive syntax. The case of all JavaScript identifiers is important.
The variables lastName and lastname are not the same thing:
var lastname, lastName;lastName = 'Doe';lastname = 'Peterson';
VAR or Var are not interpreted by JavaScript as the keyword var.
JavaScript and Camel Case
Programmers have traditionally used a variety of methods to combine numerous words into a single variable name:
Hyphens:
Phrases with hyphens: first-name, last-name, master-card, inter-city In JavaScript, hyphens are not permitted. They're only for subtractions.
Underscore Place an underscore next to first name, last name, master card, inter city.
Upper Camel Case (Pascal Case). FirstName, LastName, MasterCard, InterCity.
Lower Camel Case: Camel case, which begins with a lowercase letter, is commonly used by JavaScript programmers: firstName, lastName, masterCard, interCity.
JavaScript Character Set
The Unicode character set is used by JavaScript. Unicode encompasses (nearly) all of the world's characters, punctuations, and symbols.
JavaScript comments: JavaScript comments can be used to explain and improve the readability of JavaScript code.
Single Line Comments: // denotes the beginning of a single-line comment. JavaScript ignores any text between // and the end of the line (will not be executed). Each code line in this example is preceded by a single-line comment:
Code:
// Change heading:document.getElementById('myH').innerHTML = 'My First Page';// Change paragraph:document.getElementById('myP').innerHTML = 'My first paragraph.';
Multi-line Comments: Comments that span multiple lines begin with / and conclude with /. JavaScript will ignore any text between / and /.
Code:
/*The code below will changethe heading with id = "myH"and the paragraph with id = "myP"in my web page:*/document.getElementById('myH').innerHTML = 'My First Page';document.getElementById('myP').innerHTML = 'My first paragraph.';
To illustrate the code in this example, a multi-line comment (comment block) is used. Single-line comments are the most prevalent. For formal documentation, block comments are frequently utilized. Preventing Execution with Comments Code testing can benefit from the use of comments to avoid code execution. When you put // in front of a code line, it turns it into a comment instead of an executable line.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts