Tutorial: Javascript Array Iteration
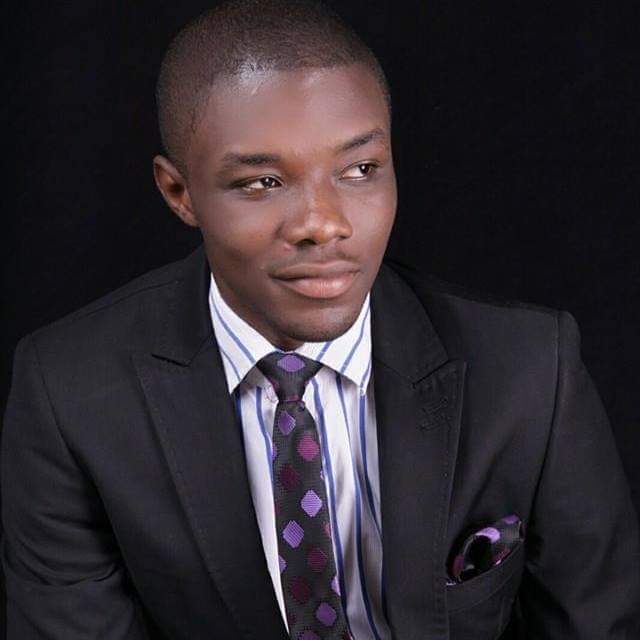
By Emmanuel Chinonso
Web Developer
JavaScript Array Iteration Methods
Array iteration methods run through all of the items inside the array.
Array.forEach() : For each array element, the forEach() method calls a function (a callback function).
JavaScript Code:
var txt = '';var numbers = [45, 4, 9, 16, 25];numbers.forEach(myFunction);
function myFunction(value, index, array) { txt = txt + value + '<br>';}
It's worth noting that the function requires three arguments:
- The value of the item
- The index of the item
- The array itself
Only the value argument is used in the preceding example. The following is a rewrite of the example:
JavaScript Code:
var txt = '';var numbers = [45, 4, 9, 16, 25];numbers.forEach(myFunction);
function myFunction(value) { txt = txt + value + '<br>';}
In all browsers, Array.forEach() is supported.
Array.map() : By applying a function to each array element, the map() method builds a new array. For array items with no values, the map() method does not execute the function. The original array is not changed by the map() operation. Each array value is multiplied by two in this code:
JavaScript Code:
var numbers1 = [45, 4, 9, 16, 25];var numbers2 = numbers1.map(myFunction);
function myFunction(value, index, array) { return value * 2;}
It's worth noting that the function requires three arguments:
- The value of the item
- The index of the item
- The array itself
When only the value parameter is used in a callback function, the index and array parameters can be omitted:
JavaScript Code:
var numbers1 = [45, 4, 9, 16, 25];var numbers2 = numbers1.map(myFunction);
function myFunction(value) { return value * 2;}
In all browsers, Array.map() is supported.
Array.filter() : The filter() method returns a new array that contains array elements that pass a test.
JavaScript Code:
var numbers = [45, 4, 9, 16, 25];var over18 = numbers.filter(myFunction);
function myFunction(value, index, array) { return value > 18;}
It's worth noting that the function requires three arguments:
- The item's worth
- The index of items
- The array in question
The index and array parameters are not used in the callback function in the code above, therefore they can be omitted:
JavaScript Code:
var numbers = [45, 4, 9, 16, 25];var over18 = numbers.filter(myFunction);
function myFunction(value) { return value > 18;}
In all browsers, Array.filter() is supported.
Array.reduce() : The reduce() method applies a function to each array element in order to generate (reduce) a single value. The reduce() method works in the array from left to right. ReduceRight is another option (). The original array is not reduced by the reduce() method.
The total of all the numbers in an array is found with this code:
JavaScript Code:
var numbers1 = [45, 4, 9, 16, 25];var sum = numbers1.reduce(myFunction);
function myFunction(total, value, index, array) { return total + value;}
It's worth noting that the function requires four arguments:
- The sum (the initial value minus the value previously returned)
- The item's worth
- The index of items
- The array in question
The index and array parameters are not used in the code above. It can be rephrased as:
JavaScript Code:
var numbers1 = [45, 4, 9, 16, 25];var sum = numbers1.reduce(myFunction);
function myFunction(total, value) { return total + value;}
An initial value can be passed to the reduce() method:
JavaScript Code:
var numbers1 = [45, 4, 9, 16, 25];var sum = numbers1.reduce(myFunction, 100);
function myFunction(total, value) { return total + value;}
In all browsers, Array.reduce() is supported.
Array.reduceRight() : The reduceRight() method applies a function to each array element in order to generate (reduce) a single value. ReduceRight() works in the array from right to left. reduce() is another option. The original array is not reduced by the reduceRight() method.
JavaScript Code:
var numbers1 = [45, 4, 9, 16, 25];var sum = numbers1.reduceRight(myFunction);
function myFunction(total, value, index, array) { return total + value;}var numbers1 = [45, 4, 9, 16, 25];var sum = numbers1.reduceRight(myFunction);
function myFunction(total, value, index, array) { return total + value;}
It's worth noting that the function requires four arguments:
- The sum (the initial value minus the value previously returned)
- The item's worth
- The index of items
- The array in question
The index and array parameters are not used in the preceding example. It can be rephrased as:
JavaScript Code:
var numbers1 = [45, 4, 9, 16, 25];var sum = numbers1.reduceRight(myFunction);
function myFunction(total, value) { return total + value;}
In all browsers, Array.reduceRight() is supported.
Array.every() : The every() method examines all array values and determines whether they pass a test.
var numbers = [45, 4, 9, 16, 25];var allOver18 = numbers.every(myFunction);
function myFunction(value, index, array) { return value > 18;}
It's worth noting that the function requires three parameters:
- The array itself
- The item value
- The item index The additional parameters can be omitted when a callback function uses only the first parameter (value):
JavaScript Code:
var numbers = [45, 4, 9, 16, 25];var allOver18 = numbers.every(myFunction);
function myFunction(value) { return value > 18;}
In all browsers, Array. every() is supported.
Array.some() : The some() method determines whether or not a set of array values pass a test.
JavaScript Code:
var numbers = [45, 4, 9, 16, 25];var someOver18 = numbers.some(myFunction);
function myFunction(value, index, array) { return value > 18;}
It's worth noting that the function requires three arguments:
- The value of the item
- The index of the item
- The array itself
Except for Internet Explorer 8 and earlier, all browsers implement Array.some().
Syntax
array.indexOf(item, start);
- item Required. The thing you're looking for.
- start Optional. Where to start the search. Negative values will begin counting from the end and search to the end at the provided point.
Array.indexOf() returns -1 if the object isn't discovered. If the item appears more than once, it returns the earliest occurrence's location.
Array.lastIndexOf() : Array.lastIndexOf() is similar to Array.indexOf(), except it returns the position of the supplied element's last occurrence. Find the item "Apple" in an array.
JavaScript Code:
var fruits = ['Apple', 'Orange', 'Apple', 'Mango'];var a = fruits.lastIndexOf('Apple');
Except for Internet Explorer 8 and earlier, all browsers support Array.lastIndexOf().
Syntax
array.lastIndexOf(item, start);
- item Required. The item to search for
- start Optional. Where to begin the search. Negative values will begin counting from the end and search to the beginning at the provided point.
Array.find() : The value of the first array member that passes a test function is returned by the find() method.
This example looks for (and returns the value of) the first element larger than 18:
Example
var numbers = [4, 9, 16, 25, 29];var first = numbers.find(myFunction);
function myFunction(value, index, array) { return value > 18;}
It's worth noting that the function requires three arguments:
- The value of the item
- The index of the item
- The array itself
In older browsers, Array.find() isn't supported.
Array.findIndex() : The index of the first array member that passes a test function is returned by the findIndex() method.
JavaScript Code:
var numbers = [4, 9, 16, 25, 29];var first = numbers.findIndex(myFunction);function myFunction(value, index, array) { return value > 18;}
It's worth noting that the function requires three arguments:
- The value of the item
- The index of the item
- The array itself
In older browsers, Array.findIndex() isn't supported.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts