Tutorial: Javascript Arrow Functions
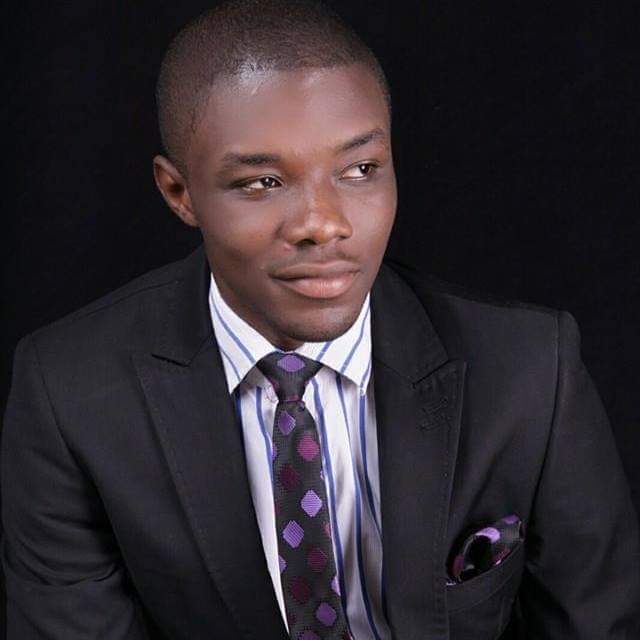
By Emmanuel Chinonso
Web Developer
JavaScript Arrow Function
In ES6, the arrow functions were introduced. Using arrow functions, we may create shorter function syntax:
Before:
hello = function() { return 'Hello World!';};
With Arrow Function:
hello = () => { return 'Hello World!';};
It is becoming shorter! If the function just has one statement that returns a value, the braces and the return keyword can be removed:
Arrow Functions Return Value by Default:
hello = () => 'Hello World!';
This only works if the function only contains one statement. If there are any parameters, they must be passed inside the parentheses:
Arrow Function With Parameters:
hello = val => 'Hello ' + val;
In fact, if there is only one parameter, the parenthesis can be omitted: Arrow Function Without Parentheses:
hello = val => 'Hello ' + val;
What About this?
The way arrow functions handle this differs from how ordinary functions handle it. In brief, there is no binding of this with arrow functions. "This" keyword was used in ordinary functions to represent the object that called the function, which could be a window, a document, a button, or anything else.
"This" keyword always reflects the object that specified the arrow function when using arrow functions. Let's look at two codes to see what the differences are. Both JavaScript Codes invoke the same procedure twice: once when the page loads and again when the user clicks a button. The first code shows how to use a standard function, while the second shows how to utilize an arrow function.
Because the window object is the "owner" of the function, the first example produces two different objects (window and button), while the second example returns the window object twice. This represents the object that calls the function in the case of a normal function:
JavaScript Code:
// Regular Function:hello = function() { document.getElementById('demo').innerHTML += this;};// The window object calls the function:window.addEventListener('load', hello);// A button object calls the function:document.getElementById('btn').addEventListener('click', hello);
With an arrow function this represents the owner of the function:
JavaScript Code:
// Arrow Function:hello = () => { document.getElementById('demo').innerHTML += this;};
// The window object calls the function:window.addEventListener('load', hello);
// A button object calls the function:document.getElementById('btn').addEventListener('click', hello);
When working with functions, keep these distinctions in mind. If the behavior of ordinary functions isn't what you're looking for, utilize arrow functions instead.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts