How To Use Tailwind CSS In React.
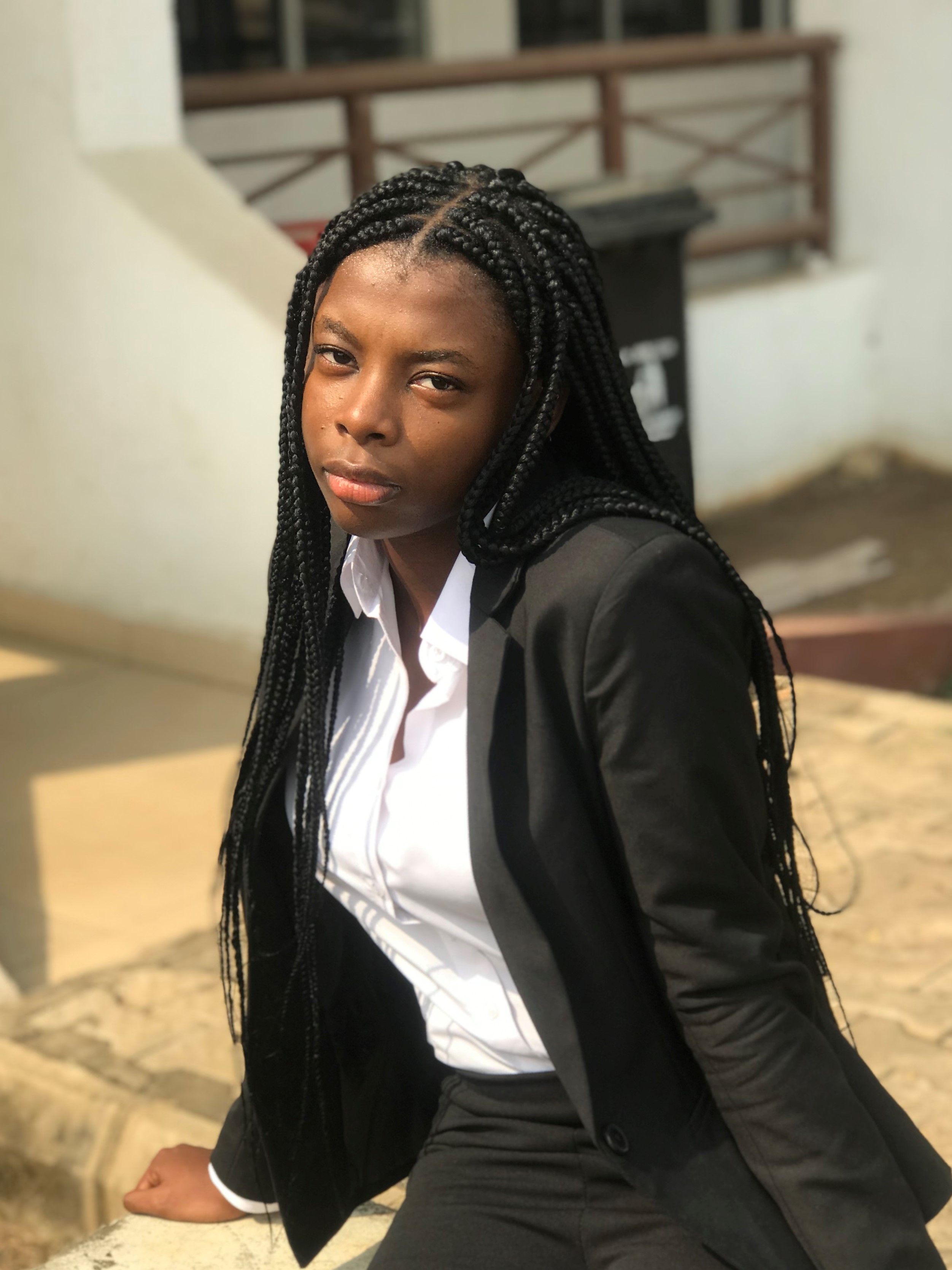
By Amarachi Iheanacho
Technical Writer
Tailwind React
Tailwind CSS is a popular utility-first CSS framework that provides a comprehensive set of pre-designed classes to help you quickly build beautiful and responsive user interfaces. When combined with React, a powerful JavaScript library for building user interfaces, you can create stunning and functional web applications with ease. In this blog post, we will explore how to use Tailwind CSS in React and highlight some best practices along the way.
Table of Content
- Prerequisites
- Getting Started
- Step 1: Create a React Project
- Step 2: Install Tailwind CSS
- Step 3: Create Tailwind Configuration Files
- Step 4: Configure tailwind.config.js
- Step 5: Create Stylesheet Entry Point
- Step 6: Import Tailwind CSS Styles
- Step 7: Start Your React Project
- Conclusion
Prerequisites
Before we dive into using Tailwind CSS in React, make sure you have the following installed:
- Node.js: This will provide the npm package manager, which we will use to install dependencies.
- Create React App: A convenient tool for setting up a new React project with all the necessary configurations.
If you don't have these installed, you can follow the official documentation for Node.js and Create React App to get started.
Getting Started
Once you have the prerequisites set up, follow these steps to start using Tailwind CSS in your React project:
Step 1: Create a New React Project
Open your terminal and navigate to the directory where you want to create your project. Run the following command to create a new React project using Create React App:
In this step-by-step guide, we'll walk through the process of integrating Tailwind CSS into a React project.
npx create-react-app my-tailwind-react-app
Replace "my-tailwind-react-app" with your desired project name.
Step 2: Install Tailwind CSS
Navigate to your project directory:
cd my-tailwind-react-app
Install Tailwind CSS and its dependencies using npm:
npm install tailwindcss postcss autoprefixer
Step 3: Create Tailwind Configuration Files
Generate the configuration files for Tailwind CSS:
npx tailwindcss init -p
This command creates a tailwind.config.js file and a postcss.config.js file in your project root.
Step 4: Configure tailwind.config.js
Open the tailwind.config.js
file in your code editor. Customize the configuration based on your project requirements. For example:
// tailwind.config.jsmodule.exports = { // ... other configurations theme: { extend: {}, }, plugins: [],}
Step 5: Create Stylesheet Entry Point
Create a new CSS file, for example, src/styles/tailwind.css, and include the following:
/* src/styles/tailwind.css */@import 'tailwindcss/base';@import 'tailwindcss/components';@import 'tailwindcss/utilities';
Step 6: Import Tailwind CSS Styles
Import the "src/styles/tailwind.css" file into your "src/index.css":
/* src/index.css *//* ... other imports */import './styles/tailwind.css';
Step 7: Start Your React Project
Now that everything is set up, start your React development server:
npm start
Visit http://localhost:3000 in your browser, and you should see your React project with Tailwind CSS styles applied.
Step 8: Using Tailwind CSS Classes in React Components
We are going to create a simple React card using Tailwind CSS. To do this, we must first open our APP.js file, delete the code there, and write the code below.
Code:
function App() { return ( <section className="App h-screen w-full flex justify-center items-center bg-purple-500"> <div className="w-full max-w-md bg-white-800"> <div className="rounded-t-xl bg-white px-6 py-6 md:p-6 text-lg md:text-xl leading-8 Md:leading-8 font-semibold" > <p className="text-purple-900 text-xl md:text-xl font-black text-center pb-2"> Tailwind CSS </p>
<p className="text-indigo-700 text-base md:text-base italic font-normal text-center" > "A utility-first CSS framework for rapid UI development. " </p> </div>
<div className="flex items-center space-x-4 p-6 md:px-6 md:py-6 bg-gradient-to-tr from-purple-700 to-indigo-700 rounded-b-xl leading-6 font-semibold text-white" > <div className="flex-none w-14 h-14 bg-white rounded-full shadow-2xl items-center justify-center" > <svg height="54" preserveAspectRatio="xMidYMid" width="54" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 256 153.6" > <linearGradient id="a" x1="-2.778%" y1="32%" y2="67.556%"> <stop offset="0" stop-color="#2298bd" /> <stop offset="1" stop-color="#0ed7b5" /> </linearGradient> <path d="M128 0C93.867 0 72.533 17.067 64 51.2 76.8 34.133 91. 733 27.733 108.8 32c9.737 2.434 16.697 9.499 24.401 17. 318C145.751 62.057 160.275 76.8 192 76.8c34.133 0 55.467-17. 067 64-51.2-12.8 17.067-27.733 23.467-44.8 19.2-9.737-2. 434-16.697-9.499-24.401-17.318C174.249 14.743 159. 725 0 128 0zM64 76.8C29.867 76.8 8.533 93.867 0 128c12.8-17. 067 27.733-23.467 44.8-19.2 9.737 2.434 16.697 9.499 24. 401 17.318C81.751 138.857 96.275 153.6 128 153.6c34.133 0 55.467-17.067 64-51.2-12.8 17.067-27.733 23.467-44.8 19. 2-9.737-2.434-16.697-9.499-24.401-17.318C110.249 91.543 95.725 76.8 64 76.8z" fill="url(#a)" /> </svg> </div>
<div className="flex-auto text-base md:text-base font-thin"> Created By <br /> <span className="text-xl md:text-xl text-purple-200 font-black">Adam Wathan</span> </div> </div> </div> </section> );}
export default App;
Your browser should look similar to the image below.
Tailwind CSS
"A utility-first CSS framework for rapid UI development."
Adam Wathan
Feel free to explore the extensive list of Tailwind CSS classes to style your React components efficiently.
Conclusion
Congratulations! You have successfully set up Tailwind CSS in your React project and learned how to use its utility classes to style your components. Tailwind CSS provides a powerful and flexible way to create beautiful and responsive designs in React with minimal effort.
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts