How to create a beautiful React multiselect.
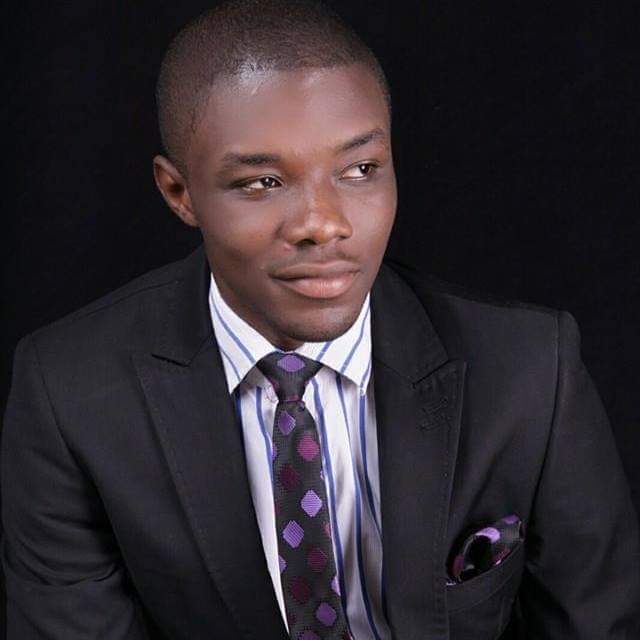
By Emmanuel Chinonso
Web Developer
React Bootstrap Multi-selects is a form control that displays a collapsable list of multiple values after the click. This is especially important in creating forms, menus, or surveys. They enable you to use the ↑
and ↓
arrow keys to navigate through options and use the↵
key to select the required option.
We will look at creating this multi-select drop-down with search today using a react library known as Contrast.
Contrast, also known as CDBReact, is a react library which is an Elegant UI kit with full bootstrap support that has reusable components for building mobile-first, responsive websites and web apps. To use the multi-select drop-down with the search, you need to download the pro version here.
Prerequisites
The react multi-select drop-down with a search would be built using React, Bootstrap, and CDBReact. You don’t need to have any previous knowledge of CDBReact, but the following are necessary:
- Basic React Knowledge
- JavaScript knowledge
- Basic Bootstrap knowledge
- NPM installed
The multi-select we are going to create in this tutorial is going to look like this.
Setup
To check that you have a node installed. To do this, run the following command in your terminal.
Code:
node-v
This should show you the current version of the node you have installed on your machine.
If you don’t have nodejs installed, download it here.
Installing node also installs npm on your PC, but you can still confirm using npm-v
. Now that we have the node installed, we can start up our React project by going to the directory of our choice and running.
Code:
npx create-react-app multiselect-app
I named the project multiselect-app
, but you can use any name of your choice.
Install CDBReact
Now, we have to install CDBReact in our project Run the following command to install CBDReact
npm install --save ./path-to-the-cdbreact-pro-tgz-file
Or using Yarn
yarn add ./path-to-the-cdbreact-pro-tgz-file
Note that we don’t need to install bootstrap or add it anywhere in our project, as CDBReact does that for us upon installation.
Multi-select dropdown with search
We can now create a file named multiselect.js
. This is where we are going to import the multi-select and container components. This is also where we will be writing our code.
Import the multi-select and container components from CDBReact
Code:
import React from "react";import { CDBMultiselect, CDBContainer } from "cdbreact-pro";
In the code below, we set up the features and styles for the multi-select.
export const Multiselect = () => {
// "showing" key:value pair optionalconst option = [ { text: "Value 1", showing: true, }, { text: "Second Value", showing: true, }, { text: "Third Value", showing: true, }, { text: "Final Value", showing: true, },];
We have the search prop included in the code below, which you can change if you wish.
return ( <CDBContainer> <CBDMultiselect search color="secondary" options={option} selected="value" /> </CDBContainer> );export default Multiselect;
After you have styled and created the multi-select with the search component, you can move to your app.js file and render the component on the App component.
Code:
import './App.css';import Multiselect from './Multiselect';import Reactdom from 'react-dom';
function App() { return ( <Router> <div className="App"> <Multiselect /> </div> </Router> );}
Reactdom.render(<App />, document.getElementById('root'))
The page we created should look like the image below
Now we have created the React Multi-select dropdown with a search using contrast.
Resources
Build modern projects using Bootstrap 5 and Contrast
Trying to create components and pages for a web app or website from
scratch while maintaining a modern User interface can be very tedious.
This is why we created Contrast, to help drastically reduce the amount of time we spend doing that.
so we can focus on building some other aspects of the project.
Contrast Bootstrap PRO consists of a Premium UI Kit Library featuring over 10000+ component variants.
Which even comes bundled together with its own admin template comprising of 5 admin dashboards and 23+ additional admin and multipurpose pages for
building almost any type of website or web app.
See a demo and learn more about Contrast Bootstrap Pro by clicking here.
Related Posts